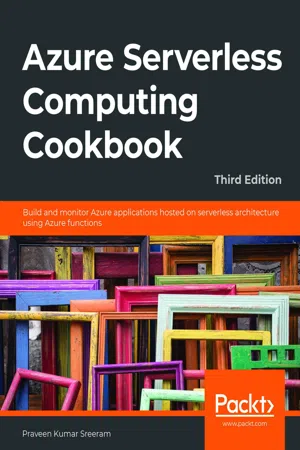
Azure Serverless Computing Cookbook
Build and monitor Azure applications hosted on serverless architecture using Azure functions, 3rd Edition
- 458 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Azure Serverless Computing Cookbook
Build and monitor Azure applications hosted on serverless architecture using Azure functions, 3rd Edition
About This Book
Discover recipes for implementing solutions to real-world business problems through serverless applications
Key Features
- Test, troubleshoot, and monitor Azure functions to deliver high-quality and reliable cloud-centric applications
- Understand Visual Studio's integrated developer experience for Azure functions
- Explore best practices for organizing and refactoring code within the Azure functions
Book Description
This third edition of Azure Serverless Computing Cookbook guides you through the development of a basic back-end web API that performs simple operations, helping you understand how to persist data in Azure Storage services. You'll cover the integration of Azure Functions with other cloud services, such as notifications (SendGrid and Twilio), Cognitive Services (computer vision), and Logic Apps, to build simple workflow-based applications.
With the help of this book, you'll be able to leverage Visual Studio tools to develop, build, test, and deploy Azure functions quickly. It also covers a variety of tools and methods for testing the functionality of Azure functions locally in the developer's workstation and in the cloud environment. Once you're familiar with the core features, you'll explore advanced concepts such as durable functions, starting with a "hello world" example, and learn about the scalable bulk upload use case, which uses durable function patterns, function chaining, and fan-out/fan-in.
By the end of this Azure book, you'll have gained the knowledge and practical experience needed to be able to create and deploy Azure applications on serverless architectures efficiently.
What you will learn
- Implement continuous integration and continuous deployment (CI/CD) of Azure functions
- Develop different event-based handlers in a serverless architecture
- Integrate Azure functions with different Azure services to develop enterprise-level applications
- Accelerate your cloud application development using Azure function triggers and bindings
- Automate mundane tasks at various levels, from development to deployment and maintenance
- Develop stateful serverless applications and self-healing jobs using durable functions
Who this book is for
If you are a cloud developer or architect who wants to build cloud-native systems and deploy serverless applications with Azure functions, this book is for you. Prior experience with Microsoft Azure core services will help you to make the most out of this book.
Frequently asked questions
Information
1. Accelerating cloud app development using Azure Functions
- Building a back-end web API using HTTP triggers
- Persisting employee details using Azure Table storage output bindings
- Saving profile picture paths to queues using queue output bindings
- Storing images in Azure Blob Storage
- Resizing an image using an ImageResizer trigger
Introduction

Figure 1.1: The key processes
- Client call to the API.
- Persist employee details using Azure Table Storage.
- Save profile picture links to queues.
- Invoke a queue trigger as soon as a message is created.
- Create the blobs in Azure Blob Storage.
- Invoke the blob trigger as soon as a blob is created.
- Resize the image and store it in Azure Blob Storage.
Building a back-end web API using HTTP triggers
Getting ready
- Refer to https://azure.microsoft.com/free/ to see how to create a free Azure account.
- Visit https://docs.microsoft.com/azure/azure-functions/functions-create-function-app-portal to learn about the step-by-step process of creating a function application, and https://docs.microsoft.com/azure/azure-functions/functions-create-first-azure-function to learn how to create a function. While creating a function, a storage account is also created to store all the files.
- Learn more about Azure Functions at https://azure.microsoft.com/services/functions/.
Note
Remember the name of the storage account, as it will be used later in other chapters. - Once the function application is created, please familiarize yourself with the basic concepts of triggers and bindings, which are at the core of how Azure Functions works. I highly recommend referring to https://docs.microsoft.com/azure/azure-functions/functions-triggers-bindings before proceeding.
Note
We'll be using C# as the programming language throughout the book. Most of the functions are developed using the Azure Functions V3 runtime. However, as of the time of writing, a few recipes were not supported in the V3 runtime. Hopefully, soon after the publication of this book, Microsoft will have made those features available for the V3 runtime as well.
How to do itā¦
- Navigate to the Function App listing page by clicking on the Function Apps menu, which is available on the left-hand side.
- Create a new function by clicking on the + icon:
Figure 1.2: Adding a new function
- You'll see the Azure Functions for .NET - getting started page, which prompts you to choose the type of tools based on your preference. For the initial few chapters, we'll use the In-portal option, which can quickly create Azure Functions right from the portal without making use of any tools. However, in the coming chapters, we'll make use of Visual Studio and Azure Functions Core Tools to create these functions:
Figure 1.3: Choosing the development environment
- In the next step, select More templates⦠and click on Finish and view templates, as shown in Figure 1.4:
Figure 1.4: Choosing More templates⦠and clicking Finish and view templates
- In the Choose a template below or go to the quickstart section, choose HTTP trigger to create a new HTTP trigger function:
Figure 1.5: The HTTP trigger template
- Provide a meaningful name. For this example, I have used RegisterUser as the name of the Azure function.
- In the Authorization level drop-down menu, choose the Anonymous option. You'll learn more about all the authorization levels in Chapter 9, Configuring security for Azure Functions:
Figure 1....
Table of contents
- Preface
- 1. Accelerating cloud app development using Azure Functions
- 2. Working with notifications using the SendGrid and Twilio services
- 3. Seamless integration of Azure Functions with Azure Services
- 4. Developing Azure Functions using Visual Studio
- 5. Exploring testing tools for Azure functions
- 6. Troubleshooting and monitoring Azure Functions
- 7. Developing reliable serverless applications using durable functions
- 8. Bulk import of data using Azure Durable Functions and Cosmos DB
- 9. Configuring security for Azure Functions
- 10. Implementing best practices for Azure Functions
- 11. Configuring serverless applications in the production environment
- 12. Implementing and deploying continuous integration using Azure DevOps
- Index