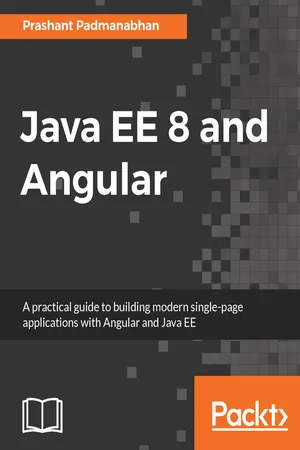
- 348 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Java EE 8 and Angular
About This Book
Learn how to build high-performing enterprise applications using Java EE powered by Angular at the frontendAbout This Book• Leverage Java EE 8 features to build robust back end for your enterprise applications• Use Angular to build a single page frontend and combine it with the Java EE backend• Practical guide filled with ample real-world examplesWho This Book Is ForThis book is for Java EE developers who would like to build modern enterprise web applications using Angular. No prior knowledge of Angular is expected.What You Will Learn• Write CDI-based code in Java EE 8 applications• Build an understanding of Microservices and what they mean in Java EE context• Use Docker to build and run a microservice application• Use configuration options to work effectively with JSON documents• Understand asynchronous task handling and writing REST API clients• Explore the fundamentals of TypeScript, which sets the foundation for working on Angular projects• Use Angular CLI to add and manage new features• Use JSON Web tokens to secure Angular applications against malicious attacksIn DetailThe demand for modern and high performing web enterprise applications is growing rapidly. No more is a basic HTML front-end enough to meet customer demands. This book will be your one stop guide to build outstanding enterprise web applications with Java EE and Angular. It will teach you how to harness the power of Java EE to build sturdy back ends while applying Angular on the front end. Your journey to building excellent web enterprise applications starts here!The book starts with a brief introduction to the fundamentals of Java EE and all the new APIs offered in the latest release. Armed with the knowledge of Java EE 8, you will go over what it's like to build an end to end application, configure database connection for JPA, and build scalable microservice using RESTful APIs running in docker containers. Taking advantage of Payara Micro capabilities, you will build an Issue Management System, which will have various features exposed as services using Java EE backend. With a detailed coverage of Angular fundamentals, the book will expand the Issue Management System by building a modern single page application frontend. Moving forward you will learn to fit both the pieces together i.e. the frontend Angular application with the backend java EE microservices. As each unit in a microservice promotes high cohesion, you will learn different ways in which independent units can be tested efficiently.Finishing off with concepts on securing your enterprise applications, this book is a hands on guide to building Modern Web Applications.Style and approachThis is a step-by-step tutorial that explains to building modern web enterprise applications.
Frequently asked questions
Information
Angular in a Nutshell
- Understanding Angular:
- Anatomy of a component
- Pipes
- Modules
- Boostrapping process
- Angular 2 and beyond:
- Angular CLI
- Managing packages
- Bootstrap dependency
- A better Hello World:
- Modules
- Components
- Handling events
- Data binding:
- One way
- Two way
- Services
- Routes
- Building a project:
- Setup and run sample
- Introduction to PrimeNG
Understanding Angular

User interface | Components |
![]() | AppComponent (Root Component) for the page, having:
|
Rendered ItemComponent UI | Description |
![]() | A child component of DashboardComponent. Represented by class ItemComponent with a header and a value. |
Anatomy of a component
Components live and die
import { Component, OnInit, OnDestroy } from '@angular/core';
class SomeComponent implements OnInit, OnDestroy {
constructor() { } // when using, new SomeComponent
ngOnInit() { } // when component is considered initialised
ngOnDestroy() { } // when component is about to be destroyed
}
- ngOnChanges(): Gets called every time any input property of component changes. Additionally, we get a map containing the change from a previous to a new value.
- ngOnInit(): Called only once and immediately after the first ngOnChanges() runs. Invocations of ngOnChanges() later does not trigger this hook again.
- ngDoCheck(): Invoked as part of the change detection process, it allows us to write our own change detection logic.
- ngAfterContentInit(): Invoked after any external content is projected in the components view.
- ngAfterContentChecked(): Invoked after checking the external content that got projected in the components view, even if no change has occurred.
- ngAfterViewInit(): Invoked after a component's views (including child views) have been fully initialized.
- ngAfterViewChecked(): Invoked after every check of a component's view.
- ngOnDestroy(): Invoked just before the component is removed. This allows for writing cleanup code such as resource release.
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- Packt Upsell
- Contributors
- Preface
- What's in Java EE 8?
- The CDI Advantage Combined with JPA
- Understanding Microservices
- Building and Deploying Microservices
- Java EE Becomes JSON Friendly
- Power Your APIs with JAXRS and CDI
- Putting It All Together with Payara
- Basic TypeScript
- Angular in a Nutshell
- Angular Forms
- Building a Real-World Application
- Connecting Angular to Java EE Microservices
- Testing Java EE Services
- Securing the Application
- Other Books You May Enjoy