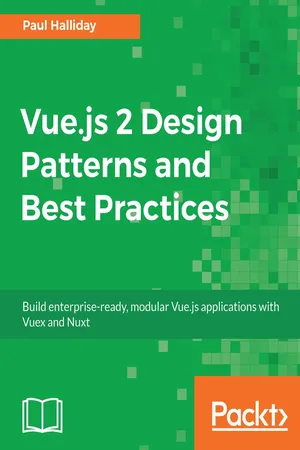
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Vue.js 2 Design Patterns and Best Practices
About This Book
Become an expert Vue developer by understanding the design patterns and component architecture of Vue.js to write clean and maintainable code.About This Bookâą Craft highly modular applications by exploring the design patterns and component architecture of Vue.jsâą Enforce a Flux-like application architecture in your Vue.js applications with Vuexâą Easy-to-follow examples that can be used to create reusable code and extensible designsWho This Book Is ForThis book targets Vue Developers who care about framework design principles and utilize commonly found design patterns in developing web applications.What You Will Learnâą Understand the theory and patterns of Vue.jsâą Build scalable and modular Vue.js applicationsâą Take advantage of Vuex for reactive state management.âą Create Single Page Applications with vue-router.âą Use Nuxt for FAST server side rendered Vue applications.âą Convert your application to a Progressive Web App (PWA) and add ServiceWorkers, offline support, and moreâą Build your app with Vue.js by following up with best practices and explore the common anti-patterns to avoidIn DetailThe book starts by comparing Vue.js with other frameworks and setting up the development environment for your application, and gradually move on to writing and styling clean, maintainable, and reusable components that can be used across your application.Further on, you'll look at common UI patterns, Vue form submission, and various modifiers such as lazy binding, number typecasting, and string trimming to create better UIs. You will also explore best practices for integrating HTTP into Vue.js applications to create an application with dynamic data.Routing is a vitally important part of any SPA, so you will focus on the Vue router and explore routing a user between multiple pages. Next, you'll also explore state management with Vuex, write testable code for your application, and create performant, server-side rendered applications with Nuxt.Towards the end, we'll look at common antipatterns to avoid, to save you from a lot of trial and error and development headaches.By the end of this book, you'll be well on your way to becoming an expert Vue developer who can leverage design patterns to efficiently architect the design of your application and write clean and maintainable code.Style and approachThis easy-to-follow practical guide will help you develop efficient Vue.js apps by following best practices and using common design patterns.
Frequently asked questions
Information
Proper Creation of Vue Projects
- Vue devtools
- Visual Studio Code extensions
- TypeScript integration
- Reactivity with RxJS
Visual Studio Code extensions
- Vetur
- Vue 2 Snippets
Vetur


Vue 2 Snippets

Vue CLI
npm install vue-cli -g
vue init webpack-simple my-vue-project

File/Folder | Description |
src/ | This folder contains all of our project code. We'll spend the majority of our time within src. |
.bablrc | This is our Babel configuration file that allows us to write ES2015 and have it appropriately transpiled. |
index.html | This is our default HTML file. |
package.json | This holds our dependencies and other project-specific metadata. |
webpack.config.js | This is our Webpack configuration file, allowing us to use .vue files, Babel, and more. |
JavaScript modules
// my-module.js
export default function add(x, y) {
return x + y
}
// my-other-module.js
import { add } from './my-other-module'
add(1, 2) // 3
Vue-loader
Table of contents
- Title Page
- Copyright and Credits
- Packt Upsell
- Contributors
- Preface
- Vue.js Principles and Comparisons
- Proper Creation of Vue Projects
- Writing Clean and Lean Code with Vue
- Vue.js Directives
- Secured Communication with Vue.js Components
- Creating Better UI
- HTTP and WebSocket Communication
- Vue Router Patterns
- State Management with Vuex
- Testing Vue.js Applications
- Optimization
- Server-Side Rendering with Nuxt
- Patterns