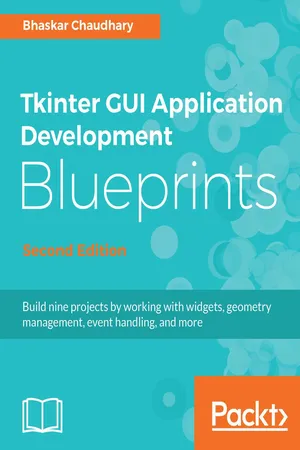
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Tkinter GUI Application Development Blueprints - Second Edition
About This Book
Geometry Management, Event Handling, and moreAbout This Book⢠A Practical, guide to learn the application of Python and GUI programming with tkinter⢠Create multiple cross-platform real-world projects by integrating host of third party libraries and tools⢠Learn to build beautiful and highly interactive user interfaces, targeting multiple devices.Who This Book Is ForThis book is for a beginner to intermediate-level Pythonists who want to build modern, cross-platform GUI applications with the amazingly powerful Tkinter. Prior knowledge of Tkinter is required.What You Will Learn⢠A Practical, guide to help you learn the application of Python and GUI programming with Tkinter⢠Create multiple, cross-platform, real-world projects by integrating a host of third-party libraries and tools⢠Learn to build beautiful and highly interactive user interfaces, targeting multiple devices.In DetailTkinter is the built-in GUI package that comes with standard Python distributions. It is a cross-platform package, which means you build once and deploy everywhere. It is simple to use and intuitive in nature, making it suitable for programmers and non-programmers alike.This book will help you master the art of GUI programming. It delivers the bigger picture of GUI programming by building real-world, productive, and fun applications such as a text editor, drum machine, game of chess, audio player, drawing application, piano tutor, chat application, screen saver, port scanner, and much more. In every project, you will build on the skills acquired in the previous project and gain more expertise. You will learn to write multithreaded programs, network programs, database-driven programs, asyncio based programming and more. You will also get to know the modern best practices involved in writing GUI apps. With its rich source of sample code, you can build upon the knowledge gained with this book and use it in your own projects in the discipline of your choice.Style and approachAn easy-to-follow guide, full of hands-on examples of real-world GUI programs. The first chapter is a must-read as it explains most of the things you need to get started with writing GUI programs with Tkinter. Each subsequent chapter is a stand-alone project that discusses some aspects of GUI programming in detail. These chapters can be read sequentially or randomly, depending on the reader's experience with Python.
Frequently asked questions
Information
Fun with Canvas
- Learning to animate with the Tkinter canvas
- Understanding the usage of polar and Cartesian coordinates on the canvas
- Implementing ordinary differential equations
- Modeling simulations given a list of formulas
- Modeling 3D graphics and studying some common transformation matrices used in 3D animation
Building a screen saver

class RandomBall:
def __init__(self, canvas):
self.canvas = canvas
self.screen_width = canvas.winfo_screenwidth()
self.screen_height = canvas.winfo_screenheight()
self.create_ball()
def create_ball(self):
self.generate_random_attributes()
self.create_oval()
def generate_random_attributes(self):
self.radius = r = randint(40, 70)
self.x_coordinate = randint(r, self.screen_width - r)
self.y_coordinate = randint(r, self.screen_height - r)
self.x_velocity = randint(6, 12)
self.y_velocity = randint(6, 12)
self.color = self.generate_random_color()
def generate_random_color(self):
r = lambda: randint(0, 0xffff)
return '#{:04x}{:04x}{:04x}'.format(r(), r(), r())
def create_oval(self):
x1 = self.x_coordinate - self.radius
y1 = self.y_coordinate - self.radius
x2 = self.x_coordinate + self.radius
y2 = self.y_coordinate + self.radius
self.ball = self.canvas.create_oval( x1, y1, x2, y2, fill=self.color,
outline=self.color)
def move_ball(self):
self.check_screen_bounds()
self.x_coordinate += self.x_velocity
self.y_coordinate += self.y_velocity
self.canvas.move(self.ball, self.x_velocity, self.y_velocity)
def check_screen_bounds(self):
r = self.radius
if not r < self.y_coordinate < self.screen_height - r:
self.y_velocity = -self.y_velocity
if not r < self.x_coordinate < self.screen_width - r:
self.x_velocity = -self.x_velocity
- Two key methods here are create_ball and move_ball. All other methods are helpers to these two methods. The __init__ method takes a canvas as a parameter and then calls the create_ball method to draw the ball on the given canvas. To move the ball around, we will explicitly need to call the move_ball method.
- The create_ball method uses the canvas.create_oval() method and move_ball uses the canvas.move(item, dx, dy) method, where dx and dy are x and y offsets for the canvas item.
- Also, note how we create a random color for the ball. Because the hexadecimal color coding system uses up to four hexadecimal digits for each of red, green, and blue, there are up to 0xffff possibilities for each color. We, therefore, create a lambda function that generates a random number from 0-0xffff, and use this function to generate three random numbers. We convert this decimal number to its two-digit equivalent hexadecimal notation using the format specifier #{:04x}{:04x}{:04x} to get a random color code for the ball.
class ScreenSaver:
balls = []
def __init__(self, number_of_balls):
self.root = Tk()
self.number_of_balls = number_of_balls
self.root.attributes('-fullscreen', True)
self.root.attributes('-alpha', 0.1)
self.root.wm_attributes('-alpha',0.1)
self.quit_on_interaction()
self.create_screensaver()
self.root.mainloop()
def create_screensaver(self):
self.create_canvas()
self.add_balls_to_canvas()
self.animate_balls()
def create_canvas(self):
self.canvas = Canvas(self.root)
self.canvas.pack(expand=1, fill=BOTH)
def add_balls_to_canvas(self):
for i in range(self.number_of_balls):
self.balls.append(RandomBall(self.canvas))
def quit_on_interaction(self):
for seq in ('<Any-KeyPress>', '<Any-Button>', '<Motion>'):
self.root.bind(seq, self.quit_screensaver)
def animate_balls(self):
for ball in self.balls:
ball.move_ball()
self.root.after(30, self.animate_balls)
def quit_screensaver(self, event):
self.root.destroy()
- The __init__ method of the ScreenSaver class takes the number of balls (number_of_balls) as its argument
- We use root.attributes ( -fullscreen, True ) to remove the enclosing frame from the parent window and make it a full-screen window.
- The quit_on_interaction method binds the root to call our quit_screensaver method in case of any interactions from the user's end.
- We then create a canvas to cover the entire screen with Canvas(self.root) with pack ( expand=1, fill=BOTH ) options to fill the entire screen.
- We create several random ball objects using the RandomBall class, passing along the Canvas widget instance as its arguments.
- We finally make a call to the animate_balls method, which uses the standard widget.after() method to keep running the animation in a loop at a regular interval of 30 milliseconds.
- To run the screen saver, we instantiate an object from our ScreenSaver class, passing the number of balls as its argument as follows: ScreenSaver(number_of_balls=18)
Table of contents
- Title Page
- Copyright and Credits
- Packt Upsell
- Contributors
- Preface
- Meet Tkinter
- Making a Text Editor
- Programmable Drum Machine
- Game of Chess
- Building an Audio Player
- Paint Application
- Piano Tutor
- Fun with Canvas
- Multiple Fun Projects
- Miscellaneous Tips
- Other Books You May Enjoy