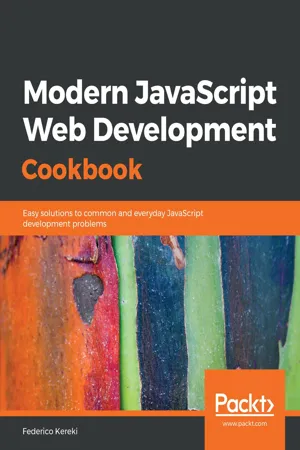
Modern JavaScript Web Development Cookbook
Easy solutions to common and everyday JavaScript development problems
Federico Kereki
- 642 Seiten
- English
- ePUB (handyfreundlich)
- Über iOS und Android verfügbar
Modern JavaScript Web Development Cookbook
Easy solutions to common and everyday JavaScript development problems
Federico Kereki
Über dieses Buch
Over 90 recipes to help you write clean code, solve common JavaScript problems, and work on popular use cases like SPAs, microservices, native mobile development with Node, React, React Native and Electron.
Key Features
- Over 90 practical recipes to help you write clean and maintainable JavaScript codes with the latest ES8
- Leverage the power of leading web frameworks like Node and React to build modern web apps
- Features comprehensive coverage of tools and techniques needed to create multi-platform apps with JavaScript
Book Description
JavaScript has evolved into a language that you can use on any platform. Modern JavaScript Web Development Cookbook is a perfect blend of solutions for traditional JavaScript development and modern areas that developers have lately been exploring with JavaScript. This comprehensive guide teaches you how to work with JavaScript on servers, browsers, mobile phones and desktops.
You will start by exploring the new features of ES8. You will then move on to learning the use of ES8 on servers (with Node.js), with the objective of producing services and microservices and dealing with authentication and CORS. Once you get accustomed to ES8, you will learn to apply it to browsers using frameworks, such as React and Redux, which interact through Ajax with services. You will then understand the use of a modern framework to develop the UI. In addition to this, development for mobile devices with React Native will walk you through the benefits of creating native apps, both for Android and iOS.
Finally, you'll be able to apply your new-found knowledge of server-side and client-side tools to develop applications with Electron.
What you will learn
- Use the latest features of ES8 and learn new ways to code with JavaScript
- Develop server-side services and microservices with Node.js
- Learn to do unit testing and to debug your code
- Build client-side web applications using React and Redux
- Create native mobile applications for Android and iOS with React Native
- Write desktop applications with Electron
Who this book is for
This book is for developers who want to explore the latest JavaScript features, frameworks, and tools for building complete mobile, desktop and web apps, including server and client-side code. You are expected to have working knowledge of JavaScript to get the most out of this book.
Häufig gestellte Fragen
Information
Using Modern JavaScript Features
- Adding types
- Working with strings
- Enhancing your code
- Defining functions
- Programming functionally
- Doing async calls compactly
- Working with objects and classes
- Organizing code in modules
- Determining a feature's availability
Introduction
- ECMAScript 1, June 1997
- ECMAScript 2, June 1998, essentially equal to the previous version
- ECMAScript 3, December 1999, adding several new functionalities
- ECMAScript 5, December 2009 (there never was an ECMAScript 4; that version was abandoned) also known as JS5
- ECMAScript 5.1, June 2011
- ECMAScript 6 (ES2015 or ES6), June 2015
- ECMAScript 7 (ES2016), June 2016
- ECMAScript 8 (ES2017), June 2017
- ECMAScript 9 (ES2018), June 2018
Adding types
Getting started
/* @flow */
/* @flow */
function foo(x: ?number): string {
if (x) {
return x;
} else {
return "some string";
}
}
console.log(foo("x"));
Error ------------------------------------------------------------------------------------- src/types_examples.js:5:16
Cannot return x because number [1] is incompatible with string [2].
2│
[1][2] 3│ function foo(x /* :?number */) /* :string */ {
4│ if (x) {
5│ return x;
6│ } else {
7│ return 'some string';
8│ }
Error------------------------------------------------------------------------------------- src/types_examples.js:12:17
Cannot call foo with 'x' bound to x because string [1] is incompatible with number [2].
[2] 3│ function foo(x /* :?number */) /* :string */ {
:
9│ }
10│
11│ // eslint-disable-next-line no-console
[1] 12│ console.log(foo('x'));
13│
How to do it...
Inhaltsverzeichnis
- Title Page
- Copyright and Credits
- Dedication
- www.PacktPub.com
- Contributors
- Preface
- Working with JavaScript Development Tools
- Using Modern JavaScript Features
- Developing with Node
- Implementing RESTful Services with Node
- Testing and Debugging Your Server
- Developing with React
- Enhancing Your Application
- Expanding Your Application
- Debugging Your Application
- Testing Your Application
- Creating Mobile Apps with React Native
- Testing and Debugging Your Mobile App
- Creating a Desktop Application with Electron
- Other Books You May Enjoy