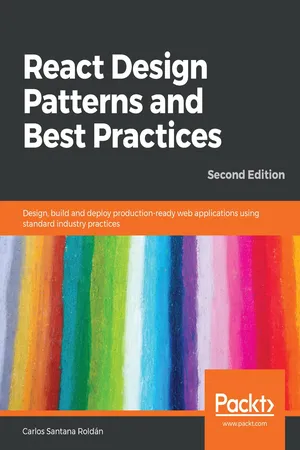
React Design Patterns and Best Practices
Design, build and deploy production-ready web applications using standard industry practices, 2nd Edition
Carlos Santana Roldan
- 350 Seiten
- English
- ePUB (handyfreundlich)
- Über iOS und Android verfügbar
React Design Patterns and Best Practices
Design, build and deploy production-ready web applications using standard industry practices, 2nd Edition
Carlos Santana Roldan
Über dieses Buch
Build modular React web apps that are scalable, maintainable and powerful using design patterns and insightful practices
Key Features
- Get familiar with design patterns in React like Render props and Controlled/uncontrolled inputs
- Learn about class/ functional, style and high order components with React
- Work through examples that can be used to create reusable code and extensible designs
Book Description
React is an adaptable JavaScript library for building complex UIs from small, detached bits called components. This book is designed to take you through the most valuable design patterns in React, helping you learn how to apply design patterns and best practices in real-life situations.
You'll get started by understanding the internals of React, in addition to covering Babel 7 and Create React App 2.0, which will help you write clean and maintainable code. To build on your skills, you will focus on concepts such as class components, stateless components, and pure components. You'll learn about new React features, such as the context API and React Hooks that will enable you to build components, which will be reusable across your applications. The book will then provide insights into the techniques of styling React components and optimizing them to make applications faster and more responsive. In the concluding chapters, you'll discover ways to write tests more effectively and learn how to contribute to React and its ecosystem.
By the end of this book, you will be equipped with the skills you need to tackle any developmental setbacks when working with React. You'll be able to make your applications more flexible, efficient, and easy to maintain, thereby giving your workflow a boost when it comes to speed, without reducing quality.
What you will learn
- Get familiar with the new React features, like context API and React Hooks
- Learn the techniques of styling and optimizing React components
- Make components communicate with each other by applying consolidate patterns
- Use server-side rendering to make applications load faster
- Write a comprehensive set of tests to create robust and maintainable code
- Build high-performing applications by optimizing components
Who this book is for
This book is for web developers who want to increase their understanding of React and apply it to real-life application development. Prior experience with React and JavaScript is assumed.
Häufig gestellte Fragen
Information
Section 1: Hello React!
- Chapter 1, Taking Your First Steps with React
- Chapter 2, Clean Up Your Code
Taking Your First Steps with React
- The difference between imperative and declarative programming
- React components and their instances, and how React uses elements to control the UI flow
- How React changes the way we build web applications, enforcing a different new concept of separation of concerns, and the reasons behind its unpopular design choice
- Why people feel the JavaScript fatigue, and what you can do to avoid the most common errors developers make when approaching the React ecosystem
Declarative programming
- Take a glass from the shelf.
- Put the glass in front of the draft.
- Pull down the handle until the glass is full.
- Pass me the glass.
toLowerCase(['FOO', 'BAR']) // ['foo', 'bar']
const toLowerCase = input => {
const output = [];
for (let i = 0; i < input.length; i++) {
output.push(input[i].toLowerCase());
}
return output;
};
const toLowerCase = input => input.map(value => value.toLowerCase());
const map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: myLatLng
});
const marker = new google.maps.Marker({
position: myLatLng,
title: 'Hello World!'
});
marker.setMap(map);
<Gmaps zoom={4} center={myLatLng}>
<Marker position={myLatLng} title="Hello world!" />
</Gmaps>
React elements
{
type: Title,
props: {
color: 'red',
children: 'Hello, Title!'
}
}
Inhaltsverzeichnis
- Title Page
- About Packt
- Copyright and Credits
- Contributors
- Preface
- Section 1: Hello React!
- Taking Your First Steps with React
- Clean Up Your Code
- Section 2: How React works
- Creating Truly Reusable Components
- Compose All the Things
- Proper Data Fetching
- Write Code for the Browser
- Section 3: Performance, Improvements and Production!
- Make Your Components Look Beautiful
- Server-Side Rendering for Fun and Profit
- Improve the Performance of Your Applications
- About Testing and Debugging
- React Router
- Anti-Patterns to be Avoided
- Deploying to Production
- Next Steps
- Other Books You May Enjoy