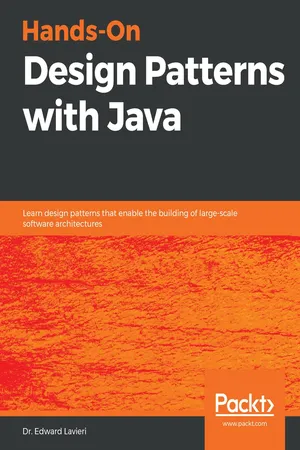
Hands-On Design Patterns with Java
Learn design patterns that enable the building of large-scale software architectures
Dr. Edward Lavieri
- 360 pages
- English
- ePUB (adapté aux mobiles)
- Disponible sur iOS et Android
Hands-On Design Patterns with Java
Learn design patterns that enable the building of large-scale software architectures
Dr. Edward Lavieri
Ă propos de ce livre
Understand Gang of Four, architectural, functional, and reactive design patterns and how to implement them on modern Java platforms, such as Java 12 and beyond
Key Features
- Learn OOP, functional, and reactive patterns for creating readable and maintainable code
- Explore architectural patterns and practices for building scalable and reliable applications
- Tackle all kinds of performance-related issues and streamline development using design patterns
Book Description
Java design patterns are reusable and proven solutions to software design problems. This book covers over 60 battle-tested design patterns used by developers to create functional, reusable, and flexible software.
Hands-On Design Patterns with Java starts with an introduction to the Unified Modeling Language (UML), and delves into class and object diagrams with the help of detailed examples. You'll study concepts and approaches to object-oriented programming (OOP) and OOP design patterns to build robust applications. As you advance, you'll explore the categories of GOF design patterns, such as behavioral, creational, and structural, that help you improve code readability and enable large-scale reuse of software. You'll also discover how to work effectively with microservices and serverless architectures by using cloud design patterns, each of which is thoroughly explained and accompanied by real-world programming solutions.
By the end of the book, you'll be able to speed up your software development process using the right design patterns, and you'll be comfortable working on scalable and maintainable projects of any size.
What you will learn
- Understand the significance of design patterns for software engineering
- Visualize software design with UML diagrams
- Strengthen your understanding of OOP to create reusable software systems
- Discover GOF design patterns to develop scalable applications
- Examine programming challenges and the design patterns that solve them
- Explore architectural patterns for microservices and cloud development
Who this book is for
If you are a developer who wants to learn how to write clear, concise, and effective code for building production-ready applications, this book is for you. Familiarity with the fundamentals of Java is assumed.
Foire aux questions
Informations
Section 1: Introducing Design Patterns
- Chapter 1, Unified Modeling Language Primer
- Chapter 2, Object-Oriented Design Patterns
Unified Modeling Language Primer
- Introducing UML
- Behavior diagrams
- Structural diagrams
Technical requirements
- Modelio, which is available at https://www.modelio.org
- Debian/Ubuntu
- macOS X
- RedHat/CentOS
- Windows 7
- Windows 8
- Windows 10
Introducing UML
Understanding behavioral UML diagrams
- Activity diagrams
- Interaction diagrams
- State machine diagrams
- Use case diagrams
Activity diagrams

Interaction diagrams

- Sequence diagram
- Communication diagram
- Timing diagram
Sequence diagrams
