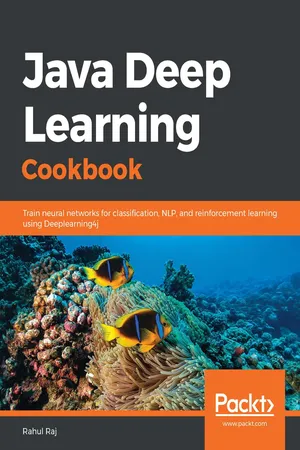
Java Deep Learning Cookbook
Train neural networks for classification, NLP, and reinforcement learning using Deeplearning4j
Rahul Raj
- 304 pagine
- English
- ePUB (disponibile sull'app)
- Disponibile su iOS e Android
Java Deep Learning Cookbook
Train neural networks for classification, NLP, and reinforcement learning using Deeplearning4j
Rahul Raj
Informazioni sul libro
Use Java and Deeplearning4j to build robust, scalable, and highly accurate AI models from scratch
Key Features
- Install and configure Deeplearning4j to implement deep learning models from scratch
- Explore recipes for developing, training, and fine-tuning your neural network models in Java
- Model neural networks using datasets containing images, text, and time-series data
Book Description
Java is one of the most widely used programming languages in the world. With this book, you will see how to perform deep learning using Deeplearning4j (DL4J) – the most popular Java library for training neural networks efficiently.
This book starts by showing you how to install and configure Java and DL4J on your system. You will then gain insights into deep learning basics and use your knowledge to create a deep neural network for binary classification from scratch. As you progress, you will discover how to build a convolutional neural network (CNN) in DL4J, and understand how to construct numeric vectors from text. This deep learning book will also guide you through performing anomaly detection on unsupervised data and help you set up neural networks in distributed systems effectively. In addition to this, you will learn how to import models from Keras and change the configuration in a pre-trained DL4J model. Finally, you will explore benchmarking in DL4J and optimize neural networks for optimal results.
By the end of this book, you will have a clear understanding of how you can use DL4J to build robust deep learning applications in Java.
What you will learn
- Perform data normalization and wrangling using DL4J
- Build deep neural networks using DL4J
- Implement CNNs to solve image classification problems
- Train autoencoders to solve anomaly detection problems using DL4J
- Perform benchmarking and optimization to improve your model's performance
- Implement reinforcement learning for real-world use cases using RL4J
- Leverage the capabilities of DL4J in distributed systems
Who this book is for
If you are a data scientist, machine learning developer, or a deep learning enthusiast who wants to implement deep learning models in Java, this book is for you. Basic understanding of Java programming as well as some experience with machine learning and neural networks is required to get the most out of this book.
Domande frequenti
Informazioni
Implementing Natural Language Processing
- Reading and loading text data
- Tokenizing data and training the model
- Evaluating the model
- Generating plots from the model
- Saving and reloading the model
- Importing Google News vectors
- Troubleshooting and tuning Word2Vec models
- Using Word2Vec for sentence classification using CNNs
- Using Doc2Vec for document classification
Technical requirements
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>deeplearning4j-nlp</artifactId>
<version>1.0.0-beta3</version>
</dependency>
Data requirements
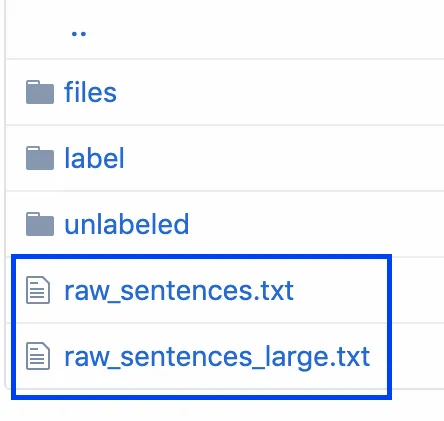
- Google News vector: https://deeplearning4jblob.blob.core.windows.net/resources/wordvectors/GoogleNews-vectors-negative300.bin.gz
- IMDB review data: http://ai.stanford.edu/~amaas/data/sentiment/aclImdb_v1.tar.gz
Reading and loading text data
Getting ready
How to do it...
- Create a sentence iterator using BasicLineIterator:
File file = new File("raw_sentences.txt");
SentenceIterator iterator = new BasicLineIterator(file);
- Create a sentence iterator using LineSentenceIterator:
File file = new File("raw_sentences.txt");
SentenceIterator iterator = new LineSentenceIterator(file);
- Create a sentence iterator using CollectionSentenceIterator:
List<String> sentences= Arrays.asList("sample text", "sample text", "sample text");
SentenceIterator iter = new Collect...
Indice dei contenuti
- Title Page
- Copyright and Credits
- Dedication
- About Packt
- Contributors
- Preface
- Introduction to Deep Learning in Java
- Data Extraction, Transformation, and Loading
- Building Deep Neural Networks for Binary Classification
- Building Convolutional Neural Networks
- Implementing Natural Language Processing
- Constructing an LSTM Network for Time Series
- Constructing an LSTM Neural Network for Sequence Classification
- Performing Anomaly Detection on Unsupervised Data
- Using RL4J for Reinforcement Learning
- Developing Applications in a Distributed Environment
- Applying Transfer Learning to Network Models
- Benchmarking and Neural Network Optimization
- Other Books You May Enjoy