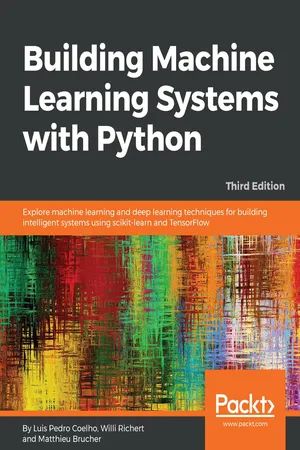
Building Machine Learning Systems with Python
Explore machine learning and deep learning techniques for building intelligent systems using scikit-learn and TensorFlow, 3rd Edition
Luis Pedro Coelho, Willi Richert, Matthieu Brucher
- 406 pagine
- English
- ePUB (disponibile sull'app)
- Disponibile su iOS e Android
Building Machine Learning Systems with Python
Explore machine learning and deep learning techniques for building intelligent systems using scikit-learn and TensorFlow, 3rd Edition
Luis Pedro Coelho, Willi Richert, Matthieu Brucher
Informazioni sul libro
Get more from your data by creating practical machine learning systems with Python
Key Features
- Develop your own Python-based machine learning system
- Discover how Python offers multiple algorithms for modern machine learning systems
- Explore key Python machine learning libraries to implement in your projects
Book Description
Machine learning allows systems to learn things without being explicitly programmed to do so. Python is one of the most popular languages used to develop machine learning applications, which take advantage of its extensive library support. This third edition of Building Machine Learning Systems with Python addresses recent developments in the field by covering the most-used datasets and libraries to help you build practical machine learning systems.
Using machine learning to gain deeper insights from data is a key skill required by modern application developers and analysts alike. Python, being a dynamic language, allows for fast exploration and experimentation. This book shows you exactly how to find patterns in your raw data. You will start by brushing up on your Python machine learning knowledge and being introduced to libraries. You'll quickly get to grips with serious, real-world projects on datasets, using modeling and creating recommendation systems. With Building Machine Learning Systems with Python, you'll gain the tools and understanding required to build your own systems, all tailored to solve real-world data analysis problems.
By the end of this book, you will be able to build machine learning systems using techniques and methodologies such as classification, sentiment analysis, computer vision, reinforcement learning, and neural networks.
What you will learn
- Build a classification system that can be applied to text, images, and sound
- Employ Amazon Web Services (AWS) to run analysis on the cloud
- Solve problems related to regression using scikit-learn and TensorFlow
- Recommend products to users based on their past purchases
- Understand different ways to apply deep neural networks on structured data
- Address recent developments in the field of computer vision and reinforcement learning
Who this book is for
Building Machine Learning Systems with Python is for data scientists, machine learning developers, and Python developers who want to learn how to build increasingly complex machine learning systems. You will use Python's machine learning capabilities to develop effective solutions. Prior knowledge of Python programming is expected.
Domande frequenti
Informazioni
Classification II – Sentiment Analysis
Sketching our roadmap
- Use this scenario as a vehicle to introduce yet another classification algorithm, Naïve Bayes
- Explain how Part Of Speech (POS) tagging works and how it can help us
- Show some more tricks from the scikit-learn toolbox that can come in handy
Fetching the Twitter data
>>> X_orig, Y_orig = load_sanders_data()
>>> classes = np.unique(Y_orig)
>>> for c in classes: print("#%s: %i" % (c, sum(Y_orig == c))) #irrelevant: 437 #negative: 448 #neutral: 1801 #positive: 391
Introducing the Naïve Bayes classifier
Getting to know the Bayes theorem
Indice dei contenuti
- Title Page
- Copyright and Credits
- Packt Upsell
- Contributors
- Preface
- Getting Started with Python Machine Learning
- Classifying with Real-World Examples
- Regression
- Classification I – Detecting Poor Answers
- Dimensionality Reduction
- Clustering – Finding Related Posts
- Recommendations
- Artificial Neural Networks and Deep Learning
- Classification II – Sentiment Analysis
- Topic Modeling
- Classification III – Music Genre Classification
- Computer Vision
- Reinforcement Learning
- Bigger Data
- Where to Learn More About Machine Learning
- Other Books You May Enjoy