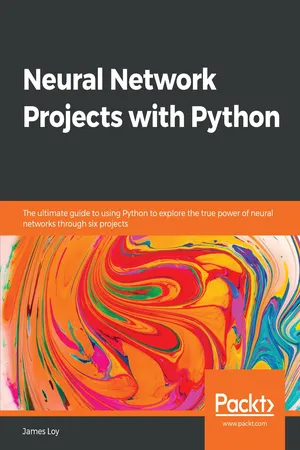
Neural Network Projects with Python
The ultimate guide to using Python to explore the true power of neural networks through six projects
James Loy
- 308 pagine
- English
- ePUB (disponibile sull'app)
- Disponibile su iOS e Android
Neural Network Projects with Python
The ultimate guide to using Python to explore the true power of neural networks through six projects
James Loy
Informazioni sul libro
Build your Machine Learning portfolio by creating 6 cutting-edge Artificial Intelligence projects using neural networks in Python
Key Features
- Discover neural network architectures (like CNN and LSTM) that are driving recent advancements in AI
- Build expert neural networks in Python using popular libraries such as Keras
- Includes projects such as object detection, face identification, sentiment analysis, and more
Book Description
Neural networks are at the core of recent AI advances, providing some of the best resolutions to many real-world problems, including image recognition, medical diagnosis, text analysis, and more. This book goes through some basic neural network and deep learning concepts, as well as some popular libraries in Python for implementing them.
It contains practical demonstrations of neural networks in domains such as fare prediction, image classification, sentiment analysis, and more. In each case, the book provides a problem statement, the specific neural network architecture required to tackle that problem, the reasoning behind the algorithm used, and the associated Python code to implement the solution from scratch. In the process, you will gain hands-on experience with using popular Python libraries such as Keras to build and train your own neural networks from scratch.
By the end of this book, you will have mastered the different neural network architectures and created cutting-edge AI projects in Python that will immediately strengthen your machine learning portfolio.
What you will learn
- Learn various neural network architectures and its advancements in AI
- Master deep learning in Python by building and training neural network
- Master neural networks for regression and classification
- Discover convolutional neural networks for image recognition
- Learn sentiment analysis on textual data using Long Short-Term Memory
- Build and train a highly accurate facial recognition security system
Who this book is for
This book is a perfect match for data scientists, machine learning engineers, and deep learning enthusiasts who wish to create practical neural network projects in Python. Readers should already have some basic knowledge of machine learning and neural networks.
Domande frequenti
Informazioni
Sentiment Analysis of Movie Reviews Using LSTM
- Sequential problems in machine learning
- NLP and sentiment analysis
- Introduction to RNNs and LSTM networks
- Analysis of the IMDb movie reviews dataset
- Word embeddings
- A step-by-step guide to building and training an LSTM network in Keras
- Analysis of our results
Technical requirements
- matplotlib 3.0.2
- Keras 2.2.4
- seaborn 0.9.0
- scikit-learn 0.20.2
$ git clone https://github.com/PacktPublishing/Neural-Network-Projects-with-Python.git
$ cd Neural-Network-Projects-with-Python
$ conda env create -f environment.yml
$ conda activate neural-network-projects-python
$ cd Chapter06
- lstm.py: This is the main code for this chapter
$ python lstm.py
Sequential problems in machine learning
- NLP, including sentiment analysis, language translation, and text prediction
- Time series predictions
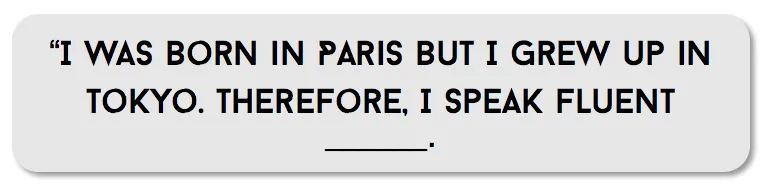
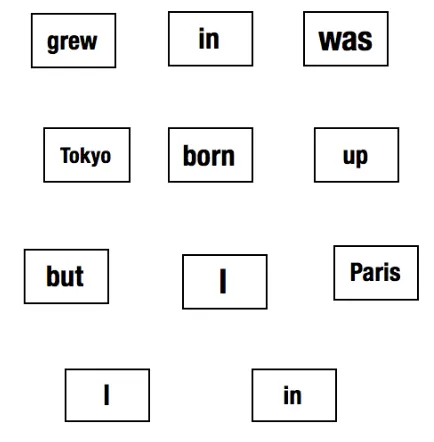
NLP and sentiment analysis
Indice dei contenuti
- Title Page
- Copyright and Credits
- Dedication
- About Packt
- Contributors
- Preface
- Machine Learning and Neural Networks 101
- Predicting Diabetes with Multilayer Perceptrons
- Predicting Taxi Fares with Deep Feedforward Networks
- Cats Versus Dogs - Image Classification Using CNNs
- Removing Noise from Images Using Autoencoders
- Sentiment Analysis of Movie Reviews Using LSTM
- Implementing a Facial Recognition System with Neural Networks
- What's Next?
- Other Books You May Enjoy