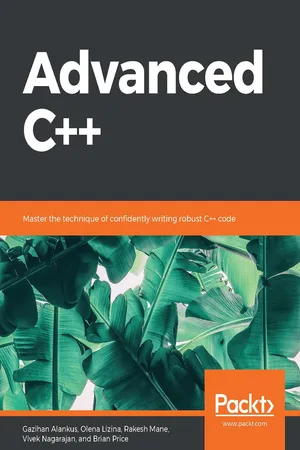
Advanced C++
Master the technique of confidently writing robust C++ code
- 762 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Advanced C++
Master the technique of confidently writing robust C++ code
About This Book
Become an expert at C++ by learning all the key C++ concepts and working through interesting exercises
Key Features
- Explore C++ concepts through descriptive graphics and interactive exercises
- Learn how to keep your development bug-free with testing and debugging
- Discover various techniques to optimize your code
Book Description
C++ is one of the most widely used programming languages and is applied in a variety of domains, right from gaming to graphical user interface (GUI) programming and even operating systems. If you're looking to expand your career opportunities, mastering the advanced features of C++ is key.
The book begins with advanced C++ concepts by helping you decipher the sophisticated C++ type system and understand how various stages of compilation convert source code to object code. You'll then learn how to recognize the tools that need to be used in order to control the flow of execution, capture data, and pass data around. By creating small models, you'll even discover how to use advanced lambdas and captures and express common API design patterns in C++. As you cover later chapters, you'll explore ways to optimize your code by learning about memory alignment, cache access, and the time a program takes to run. The concluding chapter will help you to maximize performance by understanding modern CPU branch prediction and how to make your code cache-friendly.
By the end of this book, you'll have developed programming skills that will set you apart from other C++ programmers.
What you will learn
- Delve into the anatomy and workflow of C++
- Study the pros and cons of different approaches to coding in C++
- Test, run, and debug your programs
- Link object files as a dynamic library
- Use templates, SFINAE, constexpr if expressions and variadic templates
- Apply best practice to resource management
Who this book is for
If you have worked in C++ but want to learn how to make the most of this language, especially for large projects, this book is for you. A general understanding of programming and knowledge of using an editor to produce code files in project directories is a must. Some experience with strongly typed languages, such as C and C++, is also recommended.
Frequently asked questions
Information
1. Anatomy of Portable C++ Software
Learning Objectives
- Establish the code-build-test process
- Describe the various stages of compilation
- Decipher complicated C++ type systems
- Configure projects with unit tests
- Convert source code to object code
- Write readable code and debug it
Introduction
Managing C++ Projects
The Code-Build-Test-Run Loop
Building a CMake Project

Figure 1.1: Folder structure of a sample template
Table of contents
- Preface
- 1. Anatomy of Portable C++ Software
- 2A. No Ducks Allowed – Types and Deduction
- 2B. No Ducks Allowed – Templates and Deduction
- 3. No Leaks Allowed - Exceptions and Resources
- 4. Separation of Concerns - Software Architecture, Functions, and Variadic Templates
- 5. The Philosophers' Dinner – Threads and Concurrency
- 6. Streams and I/O
- 7. Everybody Falls, It's How You Get Back Up – Testing and Debugging
- 8. Need for Speed – Performance and Optimization
- Appendix