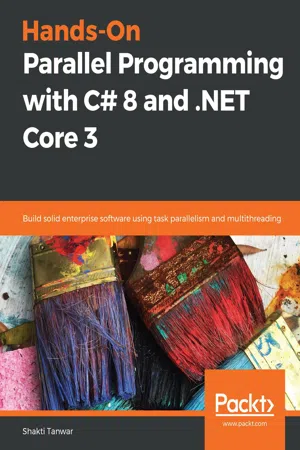
Hands-On Parallel Programming with C# 8 and .NET Core 3
Build solid enterprise software using task parallelism and multithreading
- 346 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Hands-On Parallel Programming with C# 8 and .NET Core 3
Build solid enterprise software using task parallelism and multithreading
About This Book
Enhance your enterprise application development skills by mastering parallel programming techniques in.NET and C#
Key Features
- Write efficient, fine-grained, and scalable parallel code with C# and.NET Core
- Experience how parallel programming works by building a powerful application
- Learn the fundamentals of multithreading by working with IIS and Kestrel
Book Description
In today's world, every CPU has a multi-core processor. However, unless your application has implemented parallel programming, it will fail to utilize the hardware's full processing capacity. This book will show you how to write modern software on the optimized and high-performing.NET Core 3 framework using C# 8.
Hands-On Parallel Programming with C# 8 and.NET Core 3 covers how to build multithreaded, concurrent, and optimized applications that harness the power of multi-core processors. Once you've understood the fundamentals of threading and concurrency, you'll gain insights into the data structure in.NET Core that supports parallelism. The book will then help you perform asynchronous programming in C# and diagnose and debug parallel code effectively. You'll also get to grips with the new Kestrel server and understand the difference between the IIS and Kestrel operating models. Finally, you'll learn best practices such as test-driven development, and run unit tests on your parallel code.
By the end of the book, you'll have developed a deep understanding of the core concepts of concurrency and asynchrony to create responsive applications that are not CPU-intensive.
What you will learn
- Analyze and break down a problem statement for parallelism
- Explore the APM and EAP patterns and how to move legacy code to Task
- Apply reduction techniques to get aggregated results
- Create PLINQ queries and study the factors that impact their performance
- Solve concurrency problems caused by producer-consumer race conditions
- Discover the synchronization primitives available in.NET Core
- Understand how the threading model works with IIS and Kestrel
- Find out how you can make the most of server resources
Who this book is for
If you want to learn how task parallelism is used to build robust and scalable enterprise architecture, this book is for you. Whether you are a beginner to parallelism in C# or an experienced architect, you'll find this book useful to gain insights into the different threading models supported in.NET Standard and.NET Core. Prior knowledge of C# is required to understand the concepts covered in this book.
Frequently asked questions
Information
Section 1: Fundamentals of Threading, Multitasking, and Asynchrony
- Chapter 1, Introduction to Parallel Programming
- Chapter 2, Task Parallelism
- Chapter 3, Implementing Data Parallelism
- Chapter 4, Using PLINQ
Introduction to Parallel Programming
- Basic concepts of multi-core computing, starting with an introduction to the concepts and processes related to the operating system (OS)
- Threads and the difference between multithreading and multitasking
- Advantages and disadvantages of writing parallel code and scenarios in which parallel programming is useful
Technical requirements
Preparing for multi-core computing
Processes
Some more information about the OS
- CPU speed
- Amount of RAM
- Hard disk speed (5400/7200 RPM)
- Disk type, that is, HDD or SSD
Multitasking
Hyper-threading

- A single processor with a single-core chip: One task at a time
- A single processor with an HT-enabled single-core chip: Two tasks at a time
- A single processor with a dual-core chip: Two tasks at a time
- A single processor with an HT-enabled dual-core chip: Four tasks at a time
- A single processor with a quad-core chip: Four tasks at a time
- A single processor with an HT-enabled quad-core chip: Eight tasks at a time

Flynn's taxonomy
- Single Instruction, Single Data (SISD): In this model, there is a single control unit and a single instruction stream. These syste...
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- About Packt
- Contributors
- Preface
- Section 1: Fundamentals of Threading, Multitasking, and Asynchrony
- Introduction to Parallel Programming
- Task Parallelism
- Implementing Data Parallelism
- Using PLINQ
- Section 2: Data Structures that Support Parallelism in .NET Core
- Synchronization Primitives
- Using Concurrent Collections
- Improving Performance with Lazy Initialization
- Section 3: Asynchronous Programming Using C#
- Introduction to Asynchronous Programming
- Async, Await, and Task-Based Asynchronous Programming Basics
- Section 4: Debugging, Diagnostics, and Unit Testing for Async Code
- Debugging Tasks Using Visual Studio
- Writing Unit Test Cases for Parallel and Asynchronous Code
- Section 5: Parallel Programming Feature Additions to .NET Core
- IIS and Kestrel in ASP.NET Core
- Patterns in Parallel Programming
- Distributed Memory Management
- Assessments
- Other Books You May Enjoy