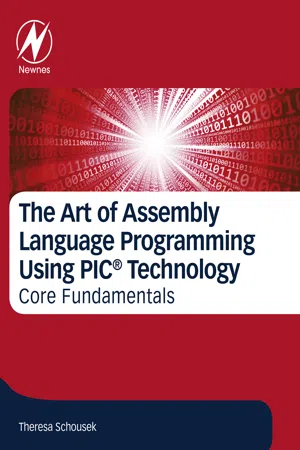
The Art of Assembly Language Programming Using PIC® Technology
Core Fundamentals
Theresa Schousek
- 458 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
The Art of Assembly Language Programming Using PIC® Technology
Core Fundamentals
Theresa Schousek
About This Book
The Art of Assembly Language Programming using PIC® Technology thoroughly covers assembly language as used in programming the PIC® Microcontroller (MCU). Using the minimal instruction set, characteristic of most PIC® products, the author elaborates on the nuances of how to execute loops. Fundamental design practices are presented based on Orr's Structured Systems Development using four logical control structures. These control structures are presented in Flowcharting, Warnier-Orr® diagrams, State Diagrams, Pseudocode, and an extended example using SysML®. Basic math instructions of Add and Subtract are presented, along with a cursory presentation of advanced math routines provided as proven Microchip® utility Application Notes.
Appendices are provided for completeness, especially for the advanced reader, including several Instruction Sets, ASCII character sets, Decimal-Binary-Hexadecimal conversion tables, and elaboration of ten 'Best Practices.' Two datasheets (one complete datasheet on the 10F20x series and one partial datasheet on the 16F88x series) are also provided in the Appendices to serve as an important reference, enabling the new embedded programmer to develop familiarity with the format of datasheets and the skills needed to assess the product datasheet for proper selection of a microcontroller family for any specific project.
The Art of Assembly Language Programming Using PIC® Technology is written for an audience with a broad variety of skill levels, ranging from the absolute beginner completely new to embedded control to the embedded C programmer new to assembly language.
With this book, you will be guided through the following areas:
- Symbols and terminology used by programmers and engineers in microcontroller applications
- Programming using assembly language through examples
- Familiarity with design and development practices
- Basics of mathematical knowledge in hexadecimal
- Resources for advanced mathematical functions
Approaches to locate resources
- Teaches how to start writing simple code, e.g., PICmicro® 10FXXX and 12FXXX
- Offers unique and novel approaches on how to add your personal touch using PICmicro® 'bread and butter' enhanced mid-range 16FXXX and 18FXXX processors
- Teaches new coding and math knowledge to help build skillsets
- Shows how to dramatically reduce product cost by achieving 100% control
- Demonstrates how to gain optimization over C programming, reduce code space, tighten up timing loops, reduce the size of microcontrollers required, and lower overall product cost
Frequently asked questions
Information
Introduction
Abstract
Keywords
Practical Applications
- • Identification tags
- • Drug tester
- • Electronic lock
- • Electronic chime
- • Pressure sensor
- • Water consumption gauge
- • Medication dispenser
- • LED flashlight
- • Intelligent power switch
- • Light dimmer
- • Fan controller
- • Smoke/CO alarm
- • Engine governor
- • Protocol handler
- • Flat iron temperature control
- • Capacitive switch
- • Irrigation controller
- • Security monitor
Why Assembly?
- From Jan Hext, in his book Programming Structures, an example of simply accessing memory to illustrate how assembly code can use registers to accomplish the task of interchanging the values of A and B. With a high level language, an additional memory location will be necessitated. A total of six memory accesses is required.
- temp := A ; Memory-to-memory requires 2 accesses
- A := B; Memory-to-memory requires 2 accesses
- B := temp ; Memory-to-memory requires 2 accesses
- With Assembly Language, registers are used which are much faster and do not require a memory access. A total of four memory accesses is required.
- R ← A ; Memory-to-register requires 1 access
- A ← B ; Memory-to-memory requires 2 accesses
- B ← R ; Register-to-memory requires 1 access
- This code may seem only minimally better than the high level language version. However, four (4) accesses versus six (6) is a savings of 33%. Some compilers do optimize and use registers. However, this is not always the case.