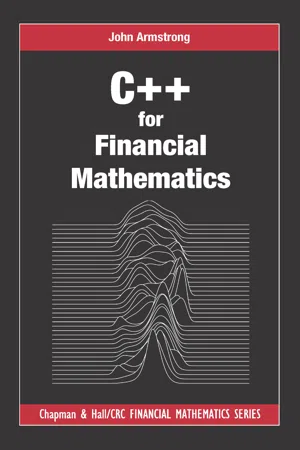
C++ for Financial Mathematics
John Armstrong
- 388 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
C++ for Financial Mathematics
John Armstrong
About This Book
If you know a little bit about financial mathematics but don't yet know a lot about programming, then C++ for Financial Mathematics is for you.
C++ is an essential skill for many jobs in quantitative finance, but learning it can be a daunting prospect. This book gathers together everything you need to know to price derivatives in C++ without unnecessary complexities or technicalities. It leads the reader step-by-step from programming novice to writing a sophisticated and flexible financial mathematics library. At every step, each new idea is motivated and illustrated with concrete financial examples.
As employers understand, there is more to programming than knowing a computer language. As well as covering the core language features of C++, this book teaches the skills needed to write truly high quality software. These include topics such as unit tests, debugging, design patterns and data structures.
The book teaches everything you need to know to solve realistic financial problems in C++. It can be used for self-study or as a textbook for an advanced undergraduate or master's level course.
Frequently asked questions
Chapter 1
Getting Started
1.1 Installing your development environment
1.1.1 For Windows
1.1.2 For Unix
g++
and make
. If they are not already installed, follow the instructions for your Unix distribution, which you should be able to readily find online. Another program you should install is gdb
, which we will discuss in Chapter 15.1.1.3 For MacOS X
1.2 Running an example program
# include <cmath >
using namespace std;
int main () {
// Interesting code starts
int principal ;
double interestRate ;
int numberOfYears ;
cout << "How much are you investing ?\n";
cin >> principal ;
cout << " What âs the annual interest rate (%)?\ n";
cin >> interestRate ;
cout << "How long for ( years )?\ n";
cin >> numberOfYears ;
double finalBalance =
pow (1.0 + interestRate * 0.01 , numberOfYears )
* principal ;
double interest = finalBalance - principal ;
cout << "You will earn ";
cout << interest ;
cout << "\n";
/* Interesting code ends */
return 0;
}
1.3 Compiling and running the code
1.3.1 Compiling on Windows
- Open Visual Studio.
- Select FileâNewâProject . . .
- Select EmptyâProject
- Enter the Name
InterestCalculator
and pressOK
. - Note the name of the folder where your project is being saved.
- Notice that to the right of the screen you have an area marked Solution Explorer inside which there is a picture of a folder marked Source Files. Right click on this and select AddâNew item. . . .
- Select the option âC++ fileâ and enter the name
main.cpp
and pressAdd
. - This creates a file called
main.cpp
which we will use to store our code. On the right-hand side of the screen you will see a text editor window where you can edit the code formain.cpp
. - Copy and paste the example code from the website4into the editor window.
- Select ProjectâInterest Calculator Properties. . ., then select LinkerâSystem and set the SubSystem to
Console (/SUBSYSTEM:CONSOLE)
using the drop down. - Press
OK
- Press
CTRL + F5
to compile and run your program
- creating new C++ source files by right clicking on the Source Files folder;
- pressing CTRL and F5 to compile and run your program.
SubSystem
.InterestCalculator/main.cpp
. This contains the code we wrote.Debug/InterestCalculator.exe
. This contains the machine code created by the compilation process. You could give someone else...
Table of contents
- Cover
- Half Title
- Title Page
- Copyright Page
- Contents
- Introduction
- 1 Getting Started
- 2 Basic Data Types and Operators
- 3 Functions
- 4 Flow of Control
- 5 Working with Multiple Files
- 6 Unit Testing
- 7 Using C++ Classes
- 8 User-Defined Types
- 9 Monte Carlo Pricing in C++
- 10 Interfaces
- 11 Arrays, Strings, and Pointers
- 12 More Sophisticated Classes
- 13 The Portfolio Class
- 14 Delta Hedging
- 15 Debugging and Development Tools
- 16 A Matrix Class
- 17 An Overview of Templates
- 18 The Standard Template Library
- 19 Function Objects and Lambda Functions
- 20 Threads
- 21 Next Steps
- A Risk-Neutral Pricing
- Bibliography
- Index