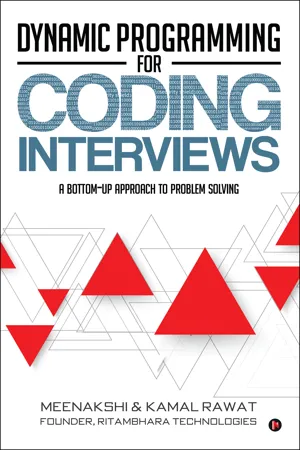
Dynamic Programming for Coding Interviews
A Bottom-Up approach to problem solving
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Dynamic Programming for Coding Interviews
A Bottom-Up approach to problem solving
About This Book
I wanted to compute 80th term of the Fibonacci series. I wrote the rampant recursive function, int fib(int n){ return (1==n || 2==n)? 1: fib(n-1) + fib(n-2);}and waited for the result. I wait⌠and wait⌠and waitâŚWith an 8GB RAM and an Intel i5 CPU, why is it taking so long? I terminated the process and tried computing the 40th term. It took about a second. I put a check and was shocked to find that the above recursive function was called 204, 668, 309 times while computing the 40th term. More than 200 million times? Is it reporting function calls or scam of some government?The Dynamic Programming solution computes 100th Fibonacci term in less than fraction of a second, with a single function call, taking linear time and constant extra memory.A recursive solution, usually, neither pass all test cases in a coding competition, nor does it impress the interviewer in an interview of company like Google, Microsoft, etc. The most difficult questions asked in competitions and interviews, are from dynamic programming. This book takes Dynamic Programming head-on. It first explain the concepts with simple examples and then deep dives into complex DP problems.
Frequently asked questions
Information
Table of contents
- Title
- Copyright
- Dedication
- Preface
- Acknowledgments
- How to Read this Book
- 1 Recursion
- 2 How it Looks in Memory
- 3 Optimal Substructure
- 4 Overlapping Subproblems
- 5 Memoization
- 6 Dynamic Programming
- 7 Top-Down v/s Bottom-Up
- 8 Strategy for DP Question
- 9 Practice Questions
- About the Author