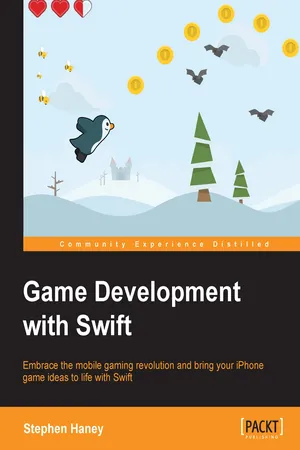
This is a test
- 224 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Game Development with Swift
Book details
Book preview
Table of contents
Citations
About This Book
If you wish to create and publish fun iOS games using Swift, then this book is for you. You should be familiar with basic programming concepts. However, no prior game development or Apple ecosystem experience is required.
Frequently asked questions
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
Both plans give you full access to the library and all of Perlego’s features. The only differences are the price and subscription period: With the annual plan you’ll save around 30% compared to 12 months on the monthly plan.
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, we’ve got you covered! Learn more here.
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Yes, you can access Game Development with Swift by Stephen Haney in PDF and/or ePUB format, as well as other popular books in Computer Science & Programming Games. We have over one million books available in our catalogue for you to explore.
Information
Game Development with Swift
Table of Contents
Game Development with Swift
Credits
About the Author
About the Reviewers
www.PacktPub.com
Support files, eBooks, discount offers, and more
Why subscribe?
Free access for Packt account holders
Preface
What this book covers
What you need for this book
Who this book is for
Conventions
Reader feedback
Customer support
Downloading the example code
Downloading the color images of this book
Errata
Piracy
Questions
1. Designing Games with Swift
Why you will love Swift
Beautiful syntax
Interoperability
Strong typing
Smart type inference
Automatic memory management
An even playing field
Are there any downsides to Swift?
Less resources
Operating system compatibility
Prerequisites
What you will learn in this book
Embracing SpriteKit
Reacting to player input
Structuring your game code
Building UI/menus/levels
Integrating with Game Center
Maximizing fun
Crossing the finish line
Further research
Marketing and monetizing your game
Making games specifically for the desktop on OSX
Setting up your development environment
Introducing Xcode
Creating our first Swift game
Navigating our project
Exploring the SpriteKit Demo
Examining the demo code
Cleaning up
Summary
2. Sprites, Camera, Actions!
Sharpening our pencils
Checkpoint 2- A
Drawing your first sprite
Building a SKSpriteNode class
Adding animation to your Toolkit
Sequencing multiple animations
Recapping your first sprite
The story on positioning
Alignment with anchor points
Adding textures and game art
Downloading the free assets
More exceptional art
Drawing your first textured sprite
Adding the bee image to your project
Loading images with SKSpriteNode
Designing for retina
The ideal asset approach
My solution for now
Hands-on with retina in SpriteKit
Organizing your assets
Exploring Images.xcassets
Collecting art into texture atlases
Updating our bee node to use the texture atlas
Iterating through texture atlas frames
Putting it all together
Centering the camera on a sprite
Creating a new world
Checkpoint 2-B
Summary
3. Mix in the Physics
Laying the foundation
Following protocol
Reinventing the bee
The icy tundra
Another way to add assets
Adding the Ground class
Tiling a texture
Running wire to the ground
A wild penguin appears!
Renovating the GameScene class
Exploring the physics system
Dropping like flies
Solidifying the ground
Checkpoint 3-A
Exploring physics simulation mechanics
Bee meets bee
Impulse or force?
Checkpoint 3-B
Summary
4. Adding Controls
Retrofitting the Player class for flight
The Beekeeper
Updating the Player class
Moving the ground
Assigning a physics body to the player
Creating a physics body shape from a texture
Polling for device movement with Core Motion
Implementing the Core Motion code
Checkpoint 4-A
Wiring up the sprite onTap events
Implementing touchesBegan in the GameScene
Larger than life
Teaching our penguin to fly
Listening for touches in GameScene
Fine-tuning gravity
Spreading your wings
Improving the camera
Pushing Pierre forward
Tracking the player's progress
Looping the ground
Checkpoint 4-B
Summary
5. Spawning Enemies, Coins, and Power-ups
Introducing the cast
Adding the power-up star
Locating the art assets
Adding the Star class
Adding a new enemy – the mad fly
Locating the enemy assets
Adding the MadFly class
Another terror – bats!
Adding the Bat class
The spooky ghost
Adding the Ghost class
Guarding the ground – adding the blade
Adding the Blade class
Adding the coins
Creating the coin classes
Organizing the project navigator
Testing the new game objects
Checkpoint 5-A
Preparing for endless flight
Summary
6. Generating a Never-Ending World
Designing levels with the SpriteKit scene editor
Separating level data from game logic
Using empty nodes as placeholders
Encounters in endless flying
Creating our first encounter
Integrating scenes into the game
Checkpoint 6-A
Spawning endless encounters
Building more encounters
Updating the EncounterManager class
Storing metadata in SKSpriteNode userData property
Wiring up EncounterManager in the GameScene class
Spawning the star power-up at random
Checkpoint 6-B
Summary
7. Implementing Collision Events
Learning the SpriteKit collision vocabulary
Collision versus contact
Physics category masks
Using category masks in Swift
Adding contact events to our game
Setting up the physics categories
Assigning categories to game objects
The player
The ground
The star power-up
Enemies
Coins
Preparing GameScene for contact events
Viewing console output
Testing our contact code
Checkpoint 7-A
Player health and damage
Animations for damage and game over
The damage animation
The game over animation
Collecting coins
The power-up star logic
Checkpoint 7-B
Summary
8. Polishing to a Shine – HUD, Parallax Backgrounds, Particles, and More
Adding a heads-up display
Parallax background layers
Adding the background assets
Implementing a background class
Wiring up backgrounds in the GameScene class
Checkpoint 8-A
Harnessing SpriteKit's particle system
Adding the circle particle asset
Creating a SpriteKit Particle File
Configuring the path particle settings
Adding the particle emitter to the game
Granting safety as the game starts
Checkpoint 8-B
Summary
9. Adding Menus and Sounds
Building the main menu
Creating the menu scene and menu nodes
Launching the main menu when the game starts
Wiring up the START GAME button
Adding the restart game menu
Extending the HUD
Wiring up GameScene for game over
Informing the GameScene class when the player dies
Implementing touch events for the restart menu
Checkpoint 9-A
Adding music and sound
Adding the sound assets to the game
Playing background music
Playing sound effects
Adding the c...
Table of contents
- Game Development with Swift