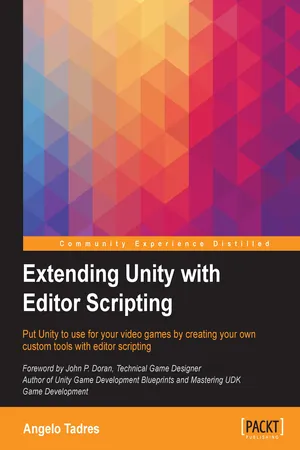
- 268 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Extending Unity with Editor Scripting
About This Book
Put Unity to use for your video games by creating your own custom tools with editor scripting
About This Book
- Acquire a good understanding of extending Unity's editor capabilities for a platformer game by using Gizmos, custom inspectors, editor windows, scriptable objects, and more
- Learn to configure and get control over your asset import pipeline using asset preprocessors
- A step-by-step, comprehensible guide to creating and customizing a build pipeline that fits the necessities of your video game development team
Who This Book Is For
This book is for anyone who has a basic knowledge of Unity programming using C# and wants to learn how to extend and create custom tools using Unity editor scripting to improve the development workflow and make video game development easier.
What You Will Learn
- Use Gizmos to create visual aids for debugging
- Extend the editor capabilities using custom inspectors, property and decorator drawers, editor windows, and handles
- Save your video game data in a persistent way using scriptable objects
- Improve the look and feel of your custom tools using GUIStyles and GUISkins
- Configure and control the asset import pipeline
- Improve the build creation pipeline
- Distribute the custom tools in your team or publish them in the Asset Store
In Detail
One of Unity's most powerful features is the extensible editor it has. With editor scripting, it is possible to extend or create functionalities to make video game development easier. For a Unity developer, this is an important topic to know and understand because adapting Unity editor scripting to video games saves a great deal of time and resources.
This book is designed to cover all the basic concepts of Unity editor scripting using a functional platformer video game that requires workflow improvement.
You will commence with the basics of editor scripting, exploring its implementation with the help of an example project, a level editor, before moving on to the usage of visual cues for debugging with Gizmos in the scene view. Next, you will learn how to create custom inspectors and editor windows and implement custom GUI. Furthermore, you will discover how to change the look and feel of the editor using editor GUIStyles and editor GUISkins. You will then explore the usage of editor scripting in order to improve the development pipeline of a video game in Unity by designing ad hoc editor tools, customizing the way the editor imports assets, and getting control over the build creation process. Step by step, you will use and learn all the key concepts while creating and developing a pipeline for a simple platform video game. As a bonus, the final chapter will help you to understand how to share content in the Asset Store that shows the creation of custom tools as a possible new business. By the end of the book, you will easily be able to extend all the concepts to other projects.
Style and approach
This book uses a step-by-step approach that will help you finish with a level editor tool, a custom configuration for the asset import pipeline, and a build pipeline totally adjusted to the video game.
Frequently asked questions
Information
Extending Unity with Editor Scripting
Table of Contents
Table of contents
- Extending Unity with Editor Scripting