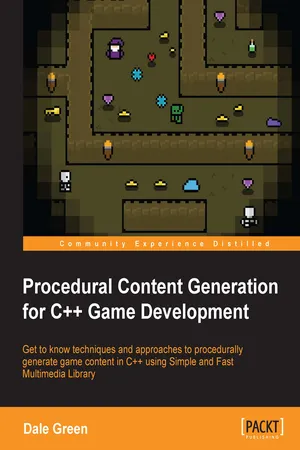
- 304 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Procedural Content Generation for C++ Game Development
About This Book
Get to know techniques and approaches to procedurally generate game content in C++ using Simple and Fast Multimedia Library
About This Book
- This book contains a bespoke Simple and Fast Multimedia Library (SFML) game engine with complete online documentation
- Through this book, you'll create games that are non-predictable and dynamic and have a high replayability factor
- Get a breakdown of the key techniques and approaches applied to a real game.
Who This Book Is For
If you are a game developer who is familiar with C++ and is looking to create bigger and more dynamic games, then this book is for you. The book assumes some prior experience with C++, but any intermediate concepts are clarified in detail. No prior experience with SFML is required.
What You Will Learn
- Discover the systems and ideology that lie at the heart of procedural systems
- Use Random number generation (RNG) with C++ data types to create random but controlled results
- Build levels procedurally with randomly located items and events
- Create dynamic game objects at runtime
- Construct games using a component-based approach
- Assemble non-predictable game events and scenarios
- Operate procedural generation to create dynamic content fast and easily
- Generate game environments for endless replayability
In Detail
Procedural generation is a growing trend in game development. It allows developers to create games that are bigger and more dynamic, giving the games a higher level of replayability. Procedural generation isn't just one technique, it's a collection of techniques and approaches that are used together to create dynamic systems and objects. C++ is the industry-standard programming language to write computer games. It's at the heart of most engines, and is incredibly powerful. SFML is an easy-to-use, cross-platform, and open-source multimedia library. Access to computer hardware is broken into succinct modules, making it a great choice if you want to develop cross-platform games with ease.
Using C++ and SFML technologies, this book will guide you through the techniques and approaches used to generate content procedurally within game development.
Throughout the course of this book, we'll look at examples of these technologies, starting with setting up a roguelike project using the C++ template. We'll then move on to using RNG with C++ data types and randomly scattering objects within a game map. We will create simple console examples to implement in a real game by creating unique and randomised game items, dynamic sprites, and effects, and procedurally generating game events. Then we will walk you through generating random game maps. At the end, we will have a retrospective look at the project.
By the end of the book, not only will you have a solid understanding of procedural generation, but you'll also have a working roguelike game that you will have extended using the examples provided.
Style and approach
This is an easy-to-follow guide where each topic is explained clearly and thoroughly through the use of a bespoke example, then implemented in a real game project.
Frequently asked questions
Information
Procedural Content Generation for C++ Game Development
Table of Contents
Table of contents
- Procedural Content Generation for C++ Game Development