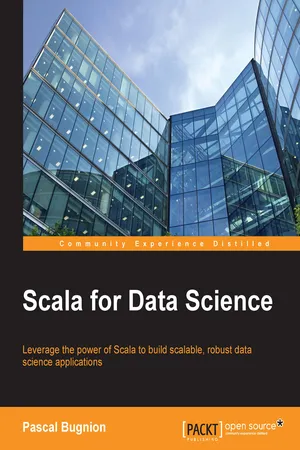
- 416 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Scala for Data Science
About This Book
Leverage the power of Scala with different tools to build scalable, robust data science applications
About This Book
- A complete guide for scalable data science solutions, from data ingestion to data visualization
- Deploy horizontally scalable data processing pipelines and take advantage of web frameworks to build engaging visualizations
- Build functional, type-safe routines to interact with relational and NoSQL databases with the help of tutorials and examples provided
Who This Book Is For
If you are a Scala developer or data scientist, or if you want to enter the field of data science, then this book will give you all the tools you need to implement data science solutions.
What You Will Learn
- Transform and filter tabular data to extract features for machine learning
- Implement your own algorithms or take advantage of MLLib's extensive suite of models to build distributed machine learning pipelines
- Read, transform, and write data to both SQL and NoSQL databases in a functional manner
- Write robust routines to query web APIs
- Read data from web APIs such as the GitHub or Twitter API
- Use Scala to interact with MongoDB, which offers high performance and helps to store large data sets with uncertain query requirements
- Create Scala web applications that couple with JavaScript libraries such as D3 to create compelling interactive visualizations
- Deploy scalable parallel applications using Apache Spark, loading data from HDFS or Hive
In Detail
Scala is a multi-paradigm programming language (it supports both object-oriented and functional programming) and scripting language used to build applications for the JVM. Languages such as R, Python, Java, and so on are mostly used for data science. It is particularly good at analyzing large sets of data without any significant impact on performance and thus Scala is being adopted by many developers and data scientists. Data scientists might be aware that building applications that are truly scalable is hard. Scala, with its powerful functional libraries for interacting with databases and building scalable frameworks will give you the tools to construct robust data pipelines.
This book will introduce you to the libraries for ingesting, storing, manipulating, processing, and visualizing data in Scala.
Packed with real-world examples and interesting data sets, this book will teach you to ingest data from flat files and web APIs and store it in a SQL or NoSQL database. It will show you how to design scalable architectures to process and modelling your data, starting from simple concurrency constructs such as parallel collections and futures, through to actor systems and Apache Spark. As well as Scala's emphasis on functional structures and immutability, you will learn how to use the right parallel construct for the job at hand, minimizing development time without compromising scalability. Finally, you will learn how to build beautiful interactive visualizations using web frameworks.
This book gives tutorials on some of the most common Scala libraries for data science, allowing you to quickly get up to speed with building data science and data engineering solutions.
Style and approach
A tutorial with complete examples, this book will give you the tools to start building useful data engineering and data science solutions straightaway
Frequently asked questions
Information
Scala for Data Science
Table of Contents
Table of contents
- Scala for Data Science