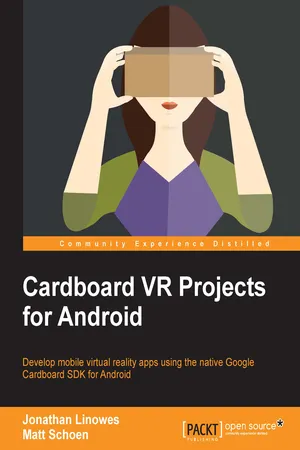
- 386 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Cardboard VR Projects for Android
About This Book
Develop mobile virtual reality apps using the native Google Cardboard SDK for Android
About This Book
- Learn how to build practical applications for Google's popular DIY VR headset
- Build a reusable VR graphics engine on top of the Cardboard Java SDK and OpenGL ES graphics libraries
- The projects in this book will showcase a different aspect of Cardboard development—from 3D rendering to handling user input
Who This Book Is For
The book is for established Android developers with a good knowledge level of Java. No prior OpenGL or graphics knowledge is required. No prior experience with Google Cardboard is expected, but those who are familiar with Cardboard and are looking for projects to expand their knowledge can also benefit from this book.
What You Will Learn
- Build Google Cardboard virtual reality applications
- Explore the ins and outs of the Cardboard SDK Java classes and interfaces, and apply them to practical VR projects
- Employ Android Studio, Android SDK, and the Java language in a straightforward manner
- Discover and use software development and Android best practices for mobile and Cardboard applications, including considerations for memory management and battery life
- Implement user interface techniques for menus and gaze-based selection within VR
- Utilize the science, psychology, mathematics, and technology behind virtual reality, especially those pertinent to mobile Cardboard VR experiences
- Understand Cardboard VR best practices including those promoted by Google Design Lab.
In Detail
Google Cardboard is a low-cost, entry-level media platform through which you can experience virtual reality and virtual 3D environments. Its applications are as broad and varied as mobile smartphone applications themselves. This book will educate you on the best practices and methodology needed to build effective, stable, and performant mobile VR applications.
In this book, we begin by defining virtual reality (VR) and how Google Cardboard fits into the larger VR and Android ecosystem. We introduce the underlying scientific and technical principles behind VR, including geometry, optics, rendering, and mobile software architecture. We start with a simple example app that ensures your environment is properly set up to write, build, and run the app. Then we develop a reusable VR graphics engine that you can build upon. And from then on, each chapter is a self-contained project where you will build an example from a different genre of application, including a 360 degree photo viewer, an educational simulation of our solar system, a 3D model viewer, and a music visualizer.
Given the recent updates that were rolled out at Google I/O 2016, the authors of Cardboard VR Projects for Android have collated some technical notes to help you execute the projects in this book with Google VR Cardboard Java SDK 0.8, released in May 2016. Refer to the article at https://www.packtpub.com/sites/default/files/downloads/GoogleVRUpdateGuideforCardbook.pdf which explains the updates to the source code of the projects.
Style and approach
This project based guide is written in a tutorial-style project format, where you will learn by doing. It is accompanied by in-depth explanations and discussions of various technologies, and provides best practices and techniques.
Frequently asked questions
Information
Cardboard VR Projects for Android
Table of Contents
Cardboard VR Projects for Android
Table of contents
- Cardboard VR Projects for Android