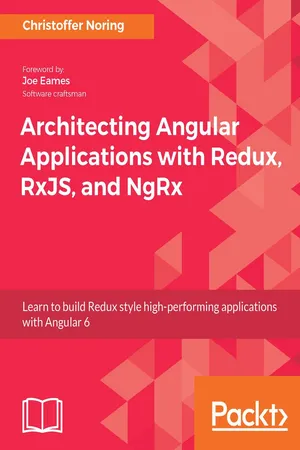
- 364 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Architecting Angular Applications with Redux, RxJS, and NgRx
About This Book
Manage state in Angular to write high performing web apps by combining the power of Flux, RxJS, and NgRxAbout This Book⢠Learn what makes an excellent Angular application architecture⢠Use Redux to write performant, consistent Angular applications⢠Incorporate Reactive Programming principles and RxJS to make it easier to develop, test, and debug your Angular applicationsWho This Book Is ForIf you have been developing Angular applications and want to dive deeper into the Angular architecture with Redux, RxJS, and NgRx to write robust web apps, then this book is for you. What You Will Learn⢠Understand the one-way data flow and Flux pattern⢠Work with functional programming and asynchronous data streams⢠Figure out how RxJS can help us address the flaws in promises⢠Set up different versions of cascading calls⢠Explore advanced operators⢠Get familiar with the Redux pattern and its principles⢠Test and debug different features of your application⢠Build your own lightweight app using Flux, Redux, and NgRxIn DetailManaging the state of large-scale web applications is a highly challenging task with the need to align different components, backends, and web workers harmoniously. When it comes to Angular, you can use NgRx, which combines the simplicity of Redux with the reactive programming power of RxJS to build your application architecture, making your code elegant and easy to reason about, debug, and test.In this book, we start by looking at the different ways of architecting Angular applications and some of the patterns that are involved in it. This will be followed by a discussion on one-way data flow, the Flux pattern, and the origin of Redux.The book introduces you to declarative programming or, more precisely, functional programming and talks about its advantages. We then move on to the reactive programming paradigm. Reactive programming is a concept heavily used in Angular and is at the core of NgRx. Later, we look at RxJS, as a library and master it. We thoroughly describe how Redux works and how to implement it from scratch. The two last chapters of the book cover everything NgRx has to offer in terms of core functionality and supporting libraries, including how to build a micro implementation of NgRx.This book will empower you to not only use Redux and NgRx to the fullest, but also feel confident in building your own version, should you need it.Style and approachThis book covers everything there is to know to get well-acquainted with Angular without bogging you down. Everything is neatly laid out under clear headings for quick consultation, giving you the information required to understand a concept immediately.
Frequently asked questions
Information
1.21 Gigawatt ā Flux Pattern Explained

- What an action and an action creator are
- How the dispatcher plays a central role in your application as a hub for messages
- State management with a store
- How to put our knowledge of Flux into practice by coding up a Flux application flow
Core concepts overview
- Action and action creators, where we set up an intention and a payload of data
- The dispatcher, our spider in the web that is able to send messages left and right
- The store, our central place for state and state management

- The application is loaded a first time, in which the data is pulled from the Store to populate the view.
- A user interaction happens in the view that leads to an intent to change something. The intent is encapsulated in an Action, and thereafter sent...
Table of contents
- Title Page
- Copyright and Credits
- Packt Upsell
- Foreword
- Contributors
- Preface
- Quick Look Back at Data Services for Simple Apps
- 1.21 Gigawatt ā Flux Pattern Explained
- Asynchronous Programming
- Functional Reactive Programming
- RxJS Basics
- Manipulating Streams and Their Values
- RxJS Advanced
- Redux
- NgRx ā Reduxing that Angular App
- NgRx ā In Depth
- Other Books You May Enjoy