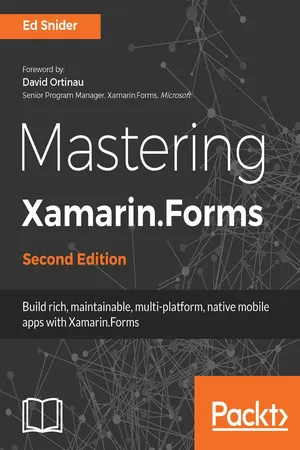
- 192 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Mastering Xamarin.Forms
About This Book
Create high-quality multi-platform native apps with Xamarin.Forms
Key Features
- Packed with real-world scenarios and solutions to help you build professional-grade mobile apps with Xamarin.Forms
- Build an effective mobile app architecture with the Xamarin.Forms toolkit
- Find out how, when, and why you should use architectural patterns and get best practices with Xamarin.Forms
Book Description
Discover how to extend and build upon the components of the Xamarin.Forms toolkit to develop an effective, robust mobile app architecture. Starting with an app built with the basics of the Xamarin.Forms toolkit, you'll go step by step through several advanced topics to create a solution architecture rich with the benefits of good design patterns and best practices.
You'll start by introducing a core separation between the app's user interface and its business logic by applying the MVVM pattern and data-binding. Then you focus on building out a layer of plugin-like services that handle platform-specific utilities such as navigation and geo-location, and on how to loosely use these services in the app with inversion of control and dependency injection. Next you connect the app to a live web-based API and set up offline synchronization. Then, you delve into testing the app logic through unit tests. Finally, you set up Visual Studio App Center for monitoring usage and bugs to gain a proactive edge on app quality.
What you will learn
- Implement the Model-View-View-Model (MVVM) pattern and data-binding in Xamarin.Forms mobile apps
- Extend the Xamarin.Forms navigation API with a custom ViewModel-centric navigation service
- Leverage the inversion of control and dependency injection patterns in Xamarin.Forms mobile apps
- Work with online and offline data in Xamarin.Forms mobile apps
- Test business logic in Xamarin.Forms mobile apps
- Use platform-specific APIs to build rich custom user interfaces in Xamarin.Forms mobile apps
- Explore how to improve mobile app quality using Visual Studio AppCenter
Who this book is for
This book is intended for C# developers who are familiar with the Xamarin platform and the Xamarin.Forms toolkit. If you have already started working with Xamarin.Forms and want to take your app to the next level with higher quality, maintainability, testability, and flexibility, then this book is for you.
Frequently asked questions
Information
Navigation
- Understanding the basics of the Xamarin.Forms navigation API
- Thinking about navigation in MVVM
- Creating a navigation service
- Updating the TripLog app to use the navigation service
The Xamarin.Forms navigation API
- PushAsync(Page page) and PushAsync(Page page, bool animated) to navigate to a new page
- PopAsync() and PopAsync(bool animated) to navigate back to the previous page, if there is one
- PushModalAsync(Page page) and PushModalAsync(Page page, bool animated) to modally display a page
- PopModalAsync() and PopModalAsync(bool animated) to dismiss the current modally displayed page
- InsertPageBefore(Page page, Page before) to insert a page before a specific page that is already in the navigation stack
- RemovePage(Page page) to remove a specific page in the navigation stack
- PopToRootAsync() and PopToRootAsync(bool animated) to navigate back to the first page and remove all others in the navigation stack
Navigation and MVVM
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- www.packtpub.com
- Foreword
- Contributors
- Preface
- Getting Started
- MVVM and Data Binding
- Navigation
- Platform Specific Services and Dependency Injection
- User Interface
- API Data Access
- Authentication
- Testing
- App Monitoring
- Other Books You May Enjoy