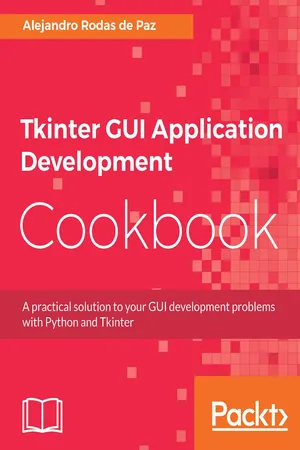
- 242 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Tkinter GUI Application Development Cookbook
About This Book
Discover solutions to all your Tkinter and Python GUI development problemsAbout This Book⢠Integrate efficient Python GUI programming techniques with Tkinter⢠Efficiently implement advanced MVC architectures in your Python GUI apps⢠Solve all your problems related to Tkinter and Python GUI developmentWho This Book Is ForThis book is for Python developers who are familiar with the basics of the language syntax, data structures, and OOP. You do not need previous experience with Tkinter or other GUI development libraries.What You Will Learn⢠Add widgets and handle user events⢠Lay out widgets within windows using frames and the different geometry managers⢠Configure widgets so that they have a customized appearance and behavior⢠Improve the navigation of your apps with menus and dialogs⢠Apply object-oriented programming techniques in Tkinter applications⢠Use threads to achieve responsiveness and update the GUI⢠Explore the capabilities of the canvas widget and the types of items that can be added to it⢠Extend Tkinter applications with the TTK (themed Tkinter) moduleIn DetailAs one of the more versatile programming languages, Python is well-known for its batteries-included philosophy, which includes a rich set of modules in its standard library; Tkinter is the library included for building desktop applications. Due to this, Tkinter is a common choice for rapid GUI development, and more complex applications can benefit from the full capabilities of this library. This book covers all of your Tkinter and Python GUI development problems and solutions.Tkinter GUI Application Development Cookbook starts with an overview of Tkinter classes and at the same time provides recipes for basic topics, such as layout patterns and event handling. Next, we cover how to develop common GUI patterns, such as entering and saving data, navigating through menus and dialogs, and performing long-running actions in the background. You will then make your apps leverage network resources effectively, perform 2D and 3D animation-related tasks, create 3D objects, and perform advanced graphical operations. Finally, this book covers using the canvas and themed widgets.By the end of the book, you will have an in-depth knowledge of Tkinter classes, and will know how to use them to build efficient and rich GUI applications.Style and approachA practical recipe-based guide that will help you find solutions to all your Tkinter and Python GUI development-related problems.
Frequently asked questions
Information
Canvas and Graphics
- Understanding the coordinate system
- Drawing lines and arrows
- Writing text on a canvas
- Adding shapes to the canvas
- Finding items by their position
- Moving canvas items
- Detecting collisions between items
- Deleting items from a canvas
- Binding events to canvas items
- Rendering a canvas into a PostScript file
Introduction
Understanding the coordinate system
How to do it...
import tkinter as tk
class App(tk.Tk):
def __init__(self):
super().__init__()
self.title("Basic canvas")
self.canvas = tk.Canvas(self, bg="white")
self.label = tk.Label(self)
self.canvas.bind("<Motion>", self.mouse_motion)
self.canvas.pack()
self.label.pack()
def mouse_motion(self, event):
x, y = event.x, event.y
text = "Mouse position: ({}, {})".format(x, y)
self.label.config(text=text)
if __name__ == "__main__":
app = App()
app.mainloop()
How it works...
def __init__(self):
# ...
self.canvas = tk.Canvas(self, bg="white")
self.label = tk.Label(self)
self.canvas.bind("<Motion>", self.mouse_motion)
- The x coordinate corresponds to the distance on the horizontal axis and increments its value when you move the cursor from left to right
- The y coordinate is the distance on the vertical axis and increments its value when you move the cursor from up to down
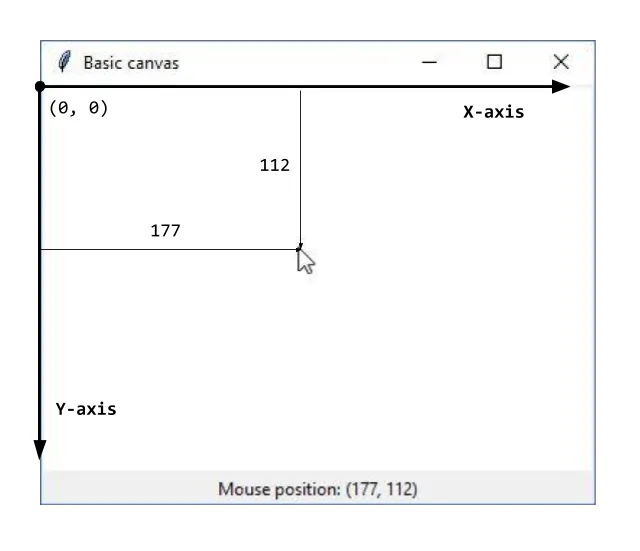
def mouse_motion(self, event):
x, y = event.x, event.y
text = "Mouse position: ({}, {})".format(x, y)
self.label.config(text=text)
There's more...
Drawing lines and arrows
Getting ready
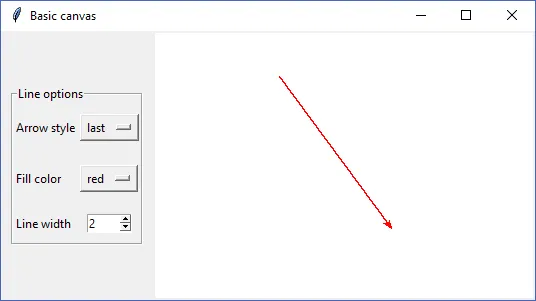
How to do it...
import tkinter as tk
class LineForm(tk.LabelFrame):
# ...
class App(tk.Tk):
def __init__(self):
super().__init__()
self.title("Basic canvas")
self.line_start = None
self.form = LineForm(self)
self.canvas = tk.Canvas(self, bg="white")
self.canvas.bind("<Button-1>", self.draw)
self.form.pack(side=tk.LEFT, padx=10, pady=10)
self.canvas.pack(side=tk.LEFT)
def draw(self, event):
x, y = event.x, event.y
if not self.line_start:
self.line_start = (x, y)
else:
x_origin, y_origin = self.line_start
self.line_start = None
line = (x_origin, y_origin, x, y)
arrow = self.form.get_arrow()
color = self.form.get_color()
width = self.form.get_width()
self.canvas.create_line(*line, arrow=arrow,
fill=color, width=width)
if __name__ == "__main__":
app = App()
app.mainloop()
How it works...
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- Packt Upsell
- Contributors
- Preface
- Getting Started with Tkinter
- Window Layout
- Customizing Widgets
- Dialogs and Menus
- Object-Oriented Programming and MVC
- Asynchronous Programming
- Canvas and Graphics
- Themed Widgets
- Other Books You May Enjoy