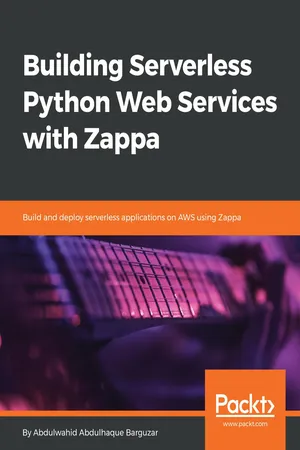
Building Serverless Python Web Services with Zappa
Build and deploy serverless applications on AWS using Zappa
- 324 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Building Serverless Python Web Services with Zappa
Build and deploy serverless applications on AWS using Zappa
About This Book
Master serverless architectures in Python and their implementation, with Zappa on three different frameworks.
Key Features
- Scalable serverless Python web services using Django, Flask, and Pyramid.
- Learn Asynchronous task execution on AWS Lambda and scheduling using Zappa.
- Implementing Zappa in a Docker container.
Book Description
Serverless applications are becoming very popular these days, not just because they save developers the trouble of managing the servers, but also because they provide several other benefits such as cutting heavy costs and improving the overall performance of the application.
This book will help you build serverless applications in a quick and efficient way. We begin with an introduction to AWS and the API gateway, the environment for serverless development, and Zappa. We then look at building, testing, and deploying apps in AWS with three different frameworks--Flask, Django, and Pyramid. Setting up a custom domain along with SSL certificates and configuring them with Zappa is also covered. A few advanced Zappa settings are also covered along with securing Zappa with AWS VPC.
By the end of the book you will have mastered using three frameworks to build robust and cost-efficient serverless apps in Python.
What you will learn
- Build, test, and deploy a simple web service using AWS CLI
- Integrate Flask-based Python applications, via AWS CLI configuration
- Design Rest APIs integrated with Zappa for Flask and Django
- Create a project in the Pyramid framework and configure it with Zappa
- Generate SSL Certificates using Amazon Certificate Manager
- Configure custom domains with AWS Route 53
- Create a Docker container similar to AWS Lambda
Who this book is for
Python Developers who are interested in learning how to develop fast and highly scalable serverless applications in Python, will find this book useful
Frequently asked questions
Information
Building a Flask Application with Zappa
- What is Flask?
- Minimal Flask application
- Configuring with Zappa
- Building, testing, and deploying on AWS Lambda
- A complete Flask Todo application
Technical requirements
- Ubuntu 16.04/macOS/Windows
- Python 3.6
- Pipenv tool
- Zappa
- Flask
- Flask Extensions
What is Flask?
Installing Flask
$ sudo apt-get install python-virtualenv

$ pip install flask
A minimal Flask application
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return 'Hello World!'
- First, we imported a Flask class.
- Next, we created an instance of the Flask class. This instance will be our WSGI application. This first argument will be the name of the module or package. Here, we created a single module, hence we used __name__. This is needed so that Flask knows where to look for templates, static, and other directories.
- Then, we used app.route as a decorator with a URL name as a parameter. This will define and map the route with the specified function.
- The function will be invoked to the HTTP request with the URL specified in the route decorator.
$ export FLASK_APP=hello_world.py
$ flask run
* Serving Flask app "flask_todo.hello_world"
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
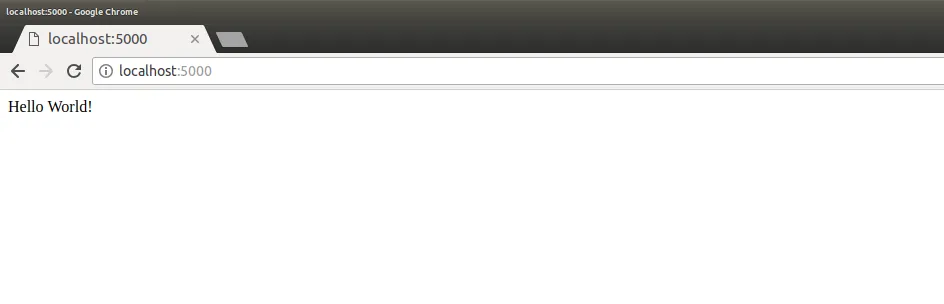
Configuring with Zappa
{
"dev": {
"app_function": "hello_world.app",
"aws_region": "ap-south-1",
"profile_name": "default",
"project_name": "flask-todo",
"runtime": "python3.6",
"s3_bucket": "zappa-yrze3w53y"
}
}
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- Packt Upsell
- Contributors
- Preface
- Amazon Web Services for Serverless
- Getting Started with Zappa
- Building a Flask Application with Zappa
- Building a Flask-Based REST API with Zappa
- Building a Django Application with Zappa
- Building a Django REST API with Zappa
- Building a Falcon Application with Zappa
- Custom Domain with SSL
- Asynchronous Task Execution on AWS Lambda
- Advanced Zappa Settings
- Securing Serverless Applications with Zappa
- Zappa with Docker
- Assessments
- Other Books You May Enjoy