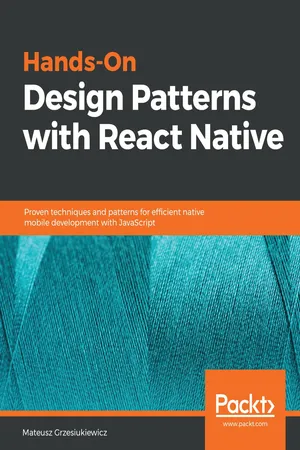
Hands-On Design Patterns with React Native
Proven techniques and patterns for efficient native mobile development with JavaScript
- 302 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Hands-On Design Patterns with React Native
Proven techniques and patterns for efficient native mobile development with JavaScript
About This Book
Learn how to write cross platform React Native code by using effective design patterns in the JavaScript world. Get to know industry standard patterns as well as situational patterns. Decouple your application with these set of "Idea patterns".
Key Features
- Mobile development in React Native should be done in a reusable way.
- Learn how to build scalable applications using JavaScript patterns that are battle tested.
- Try effective techniques on your own using over 80 standalone examples.
Book Description
React Native helps developers reuse code across different mobile platforms like iOS and Android.
This book will show you effective design patterns in the React Native world and will make you ready for professional development in big teams.
The book will focus only on the patterns that are relevant to JavaScript, ECMAScript, React and React Native. However, you can successfully transfer a lot of the skills and techniques to other languages. I call them "Idea patterns".
This book will start with the most standard development patterns in React like component building patterns, styling patterns in React Native and then extend these patterns to your mobile application using real world practical examples. Each chapter comes with full, separate source code of applications that you can build and run on your phone.
The book is also diving into architectural patterns. Especially how to adapt MVC to React environment. You will learn Flux architecture and how Redux is implementing it. Each approach will be presented with its pros and cons. You will learn how to work with external data sources using libraries like Redux thunk and Redux Saga.
The end goal is the ability to recognize the best solution for a given problem for your next mobile application.
What you will learn
- Explore the design Patterns in React Native
- Learn the best practices for React Native development
- Explore common React patterns that are highly used within React Native development
- Learn to decouple components and use dependency injection in your applications
- Explore the best ways of fetching data from the backend systems
- Learn the styling patterns and how to implement custom mobile designs
- Explore the best ways to organize your application code in big codebases
Who this book is for
The ideal target audience for this book are people eager to learn React Native design patterns who already know the basics of JavaScript. We can assume that the target audience already knows how to write Hello World in JavaScript and know what are the functions, recursive functions, JavaScript types and loops.
Frequently asked questions
Information
Styling Patterns
- Styling components in the React Native environment
- Dealing with limited style inheritance
- Using density-independent pixels
- Positioning elements with Flexbox
- Handling long text issues
- Making animations using the Animated library
- Measuring your application's speed using the Frames Per Second (FPS) metric
Technical requirements
- Simulator or Android/iOS smartphone
- Git to pull the examples: https://github.com/Ajdija/hands-on-design-patterns-with-react-native. Follow the installation instructions from the GitHub page.
How React Native styles work
- React library README (https://github.com/reactjs/react-basic/blob/master/README.md).
// Code example from React readme. Comments added for clarity.
// JavaScript pure function
// for a given input always returns the same output
function NameBox(name) {
return { fontWeight: 'bold', labelContent: name };
}
// Example with input
'Sebastian MarkbÄge' ->
{ fontWeight: 'bold', labelContent: 'Sebastian MarkbÄge' };
The style prop can be a plain old JavaScript object. (...) You can also pass an array of styles - the last style in the array has precedence, so you can use this to inherit styles.
As a component grows in complexity, it is often cleaner to use StyleSheet.create to define several styles in one place."
- React Native official documentation (https://facebook.github.io/react-native/docs/style.html).
- Using style props and passing an object with key-value pairs that represent styles.
- Using style props and passing an array of objects. Each object should contain key-value pairs that represent styles. The last style in the array has precedence. Use this mechanism to inherit styles or shadow them as you would shadow functions and variables.
- Using the StyleSheet component and its create function to create styles.
// src/ Chapter_3/ Example_1_three_ways_to_define_styles/ App.js
export default () => (
&l...
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- Packt.com
- Contributors
- Preface
- React Component Patterns
- View Patterns
- Styling Patterns
- Flux Architecture
- Store Patterns
- Data Transfer Patterns
- Navigation Patterns
- JavaScript and ECMAScript Patterns
- Elements of Functional Programming Patterns
- Managing Dependencies
- Type Checking Patterns
- Other Books You May Enjoy