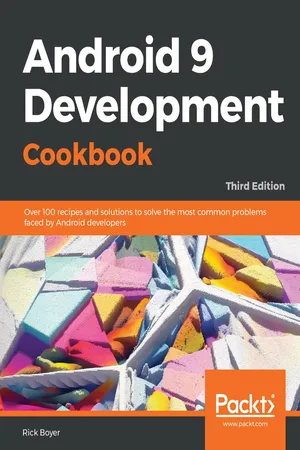
Android 9 Development Cookbook
Over 100 recipes and solutions to solve the most common problems faced by Android developers, 3rd Edition
- 464 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Android 9 Development Cookbook
Over 100 recipes and solutions to solve the most common problems faced by Android developers, 3rd Edition
About This Book
Build feature-rich, reliable Android Pie apps with the help of more than 100 proven industry standard recipes and strategies.
Key Features
- Uncover the latest features in Android 9 Pie to make your applications stand out
- Develop Android Pie applications with the latest mobile technologies, from set up to security
- Get up-to-speed with Android Studio 3 and its impressive new features
Book Description
The Android OS has the largest installation base of any operating system in the world. There has never been a better time to learn Android development to write your own applications, or to make your own contributions to the open source community! With this extensively updated cookbook, you'll find solutions for working with the user interfaces, multitouch gestures, location awareness, web services, and device features such as the phone, camera, and accelerometer. You also get useful steps on packaging your app for the Android Market. Each recipe provides a clear solution and sample code you can use in your project from the outset. Whether you are writing your first app or your hundredth, this is a book that you will come back to time and time again, with its many tips and tricks on the rich features of Android Pie.
What you will learn
- Develop applications using the latest Android framework while maintaining backward-compatibility with the support library
- Create engaging applications using knowledge gained from recipes on graphics, animations, and multimedia
- Work through succinct steps on specifics that will help you complete your project faster
- Add location awareness to your own app with examples using the latest Google Play services API
- Utilize Google Speech Recognition APIs for your app
Who this book is for
If you are new to Android development and want to take a hands-on approach to learning the framework, or if you are an experienced developer in need of clear working code to solve the many challenges in Android development, you will benefit from this book. Either way, this is a resource you'll want to keep on your desk as a quick reference to help you solve new problems as you tackle more challenging projects.
Frequently asked questions
Information
Graphics and Animation
- Scaling down large images to avoid Out of Memory exceptions
- A transition animation: Defining scenes and applying a transition
- Creating a Compass using sensor data and RotateAnimation
- Creating a slideshow with ViewPager
- Creating a Card Flip Animation with Fragments
- Creating a Zoom Animation with a Custom Transition
- Displaying an animated image (GIF/WebP) with the new ImageDecoder library
- Creating a circle image with the new ImageDecoder
Introduction
- View Animation (the original animation system): It usually requires less code but has limited animation options
- Property Animation: It's a more flexible system, allowing the animation of any property of any object
- Drawable Animation: It uses drawable resources to create frame-by-frame animations (like a movie)
- Limited aspects of what can be animated, such as scale and rotation
- Can only animate the contents of the view; it cannot change where on the screen the view is drawn (so it cannot animate moving a ball across the screen)
- Can only animate View objects
Animation blink =AnimationUtils.loadAnimation(this,R.anim.blink); view.startAnimation(blink);
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <alpha android:fromAlpha="1.0" android:toAlpha="0.0" android:background="#000000" android:interpolator="@android:anim/linear_interpolator" android:duration="100" android:repeatMode="restart" android:repeatCount="0"/> </set>
- AccelerateDecelerateInterpolator (@android:anim/accelerate_decelerate_interpolator)
- AccelerateInterpolator (@android:anim/accelerate_interpolator)
- AnticipateInterpolator (@android:anim/anticipate_interpolator)
- AnticipateOvershootInterpolator (@android:anim/anticipate_overshoot_interpolator)
- BounceInterpolator (@android:anim/bounce_interpolator)
- CycleInterpolator (@android:anim/cycle_interpolator)
- DecelerateInterpolator (@android:anim/decelerate_interpolator)
- LinearInterpolator (@android:anim/linear_interpolator)
- OvershootInterpolator (@android:anim/overshoot_interpolator)
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- About Packt
- Contributors
- Preface
- Activities
- Layouts
- Views, Widgets, and Styles
- Menus and Action Mode
- Fragments
- Home Screen Widgets, Search, and the System UI
- Data Storage
- Alerts and Notifications
- Using the Touchscreen and Sensors
- Graphics and Animation
- A First Look at OpenGL ES
- Multimedia
- Telephony, Networks, and the Web
- Location and Using Geofencing
- Getting Your App Ready for the Play Store
- Getting Started with Kotlin
- Other Books You May Enjoy