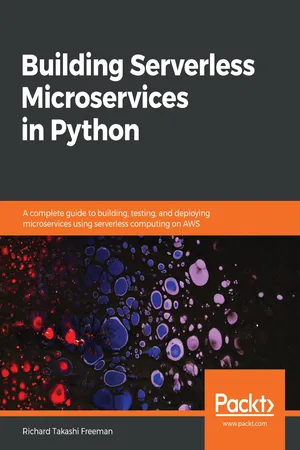
Building Serverless Microservices in Python
A complete guide to building, testing, and deploying microservices using serverless computing on AWS
- 168 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Building Serverless Microservices in Python
A complete guide to building, testing, and deploying microservices using serverless computing on AWS
About This Book
A practical guide for developing end-to-end serverless microservices in Python for developers, DevOps, and architects.
Key Features
- Create a secure, cost-effective, and scalable serverless data API
- Use identity management and authentication for a user-specific and secure web application
- Go beyond traditional web hosting to explore the full range of cloud hosting options
Book Description
Over the last few years, there has been a massive shift from monolithic architecture to microservices, thanks to their small and independent deployments that allow increased flexibility and agile delivery. Traditionally, virtual machines and containers were the principal mediums for deploying microservices, but they involved a lot of operational effort, configuration, and maintenance. More recently, serverless computing has gained popularity due to its built-in autoscaling abilities, reduced operational costs, and increased productivity.
Building Serverless Microservices in Python begins by introducing you to serverless microservice structures. You will then learn how to create your first serverless data API and test your microservice. Moving on, you'll delve into data management and work with serverless patterns. Finally, the book introduces you to the importance of securing microservices.
By the end of the book, you will have gained the skills you need to combine microservices with serverless computing, making their deployment much easier thanks to the cloud provider managing the servers and capacity planning.
What you will learn
- Discover what microservices offer above and beyond other architectures
- Create a serverless application with AWS
- Gain secure access to data and resources
- Run tests on your configuration and code
- Create a highly available serverless microservice data API
- Build, deploy, and run your serverless configuration and code
Who this book is for
If you are a developer with basic knowledge of Python and want to learn how to build, test, deploy, and secure microservices, then this book is for you. No prior knowledge of building microservices is required.
Frequently asked questions
Information
Creating Your First Serverless Data API
- Overview of security in AWS
- Securing your serverless microservice
- Building a serverless microservice data API
- Setting up Lambda security in the AWS management console
- Creating and writing to a NoSQL database called DynamoDB using AWS
- Creating and writing to a NoSQL database called DynamoDB using Python
- Creating a Lambda to query DynamoDB
- Setting up API Gateway and integrating it with a Lambda Proxy
- Connecting API Gateway, Lambda, and DynamoDB
- Cleaning-up
Overview of security in AWS
Why is security important?

- Compliance: Compliance with the law, regulations, and standards, for example, the EU General Data Protection Regulation (GDPR), the Health Information Portability and Accountability Act (HIPAA), and the Federal Trade Commission Act.
- Data integrity: If systems aren't secure, data could be stripped or tampered with, meaning you can no longer trust the customer data or financial reporting.
- Personally Identifiable Information (PII): Consumers and clients are aware of your privacy policy. Data should be securely protected, anonymized, and deleted when no longer required.
- Data availability: Data is available to authorized users, but if, for example, a natural disaster occurred in your data center, what would happen in terms of accessing data?
- Security in transit: For example, HTTPS SSLāthink of it as the padlock on your browser
- Security at rest: For example, data encryption, where only a user with a key can read the data in a data store
- Authentication: For example, a process to confirm the user or system are who they are meant to be
- Authorization: For example, permissions and control mechanisms to access specific resources
Security by design principles
- Minimize attack surface area: Every added feature is a riskāensure they are secure, for example, delete any Lambdas that are no longer being used.
- Establish secure defaults: These have defaults for every user, Identity and Access Management policy, and serverless stack component.
- Principle of least privilege: The account or service has the least amount of privilege required to perform its business processes, for example, if a Lambda only needs read access to a table, then it should have no more access than that.
- Principle of defense in depth: Have different validation layers and centralized audit controls.
- Fail securely: This ensures that if a request or transformation fails, it is still secure.
- Don't trust services: Especially third parties, external services, or libraries, for example, JavaScipt and Node.js libraries infected with malware.
- Separation of duties: Use a different role for a different task, for example, administrators should not be users or system users.
- Avoid security by obscurity: This is generally a bad idea and a weak security control. Instead of relying on the architecture or source code being secret, instead rely on other factors, such as good architecture, limiting requests, and audit controls.
- Keep security simple: Don't over-engineer; use simple architectures and design patterns.
- Fix security issues correctly: Fix issues promptly and add new tests.
AWS Identity and Access Management
JavaScript object notation
{ "firstName": "John", "lastName": "Smith", "age": 27, "address": { "city": "New York", "postalCode": "10021" }, "phoneNumbers": [ { "type": "home", "number": "212 555-1234" }, { "type": "...
Table of contents
- Title Page
- Copyright and Credits
- About Packt
- Dedication
- Contributors
- Preface
- Serverless Microservices Architectures and Patterns
- Creating Your First Serverless Data API
- Deploying Your Serverless Stack
- Testing Your Serverless Microservice
- Securing Your Microservice
- Summary and Future Work
- Other Books You May Enjoy