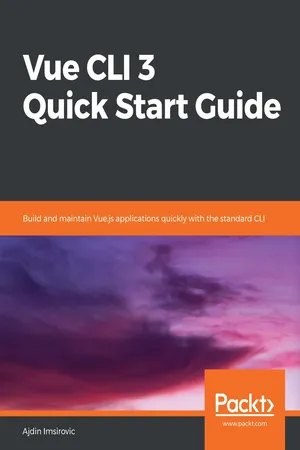
Vue CLI 3 Quick Start Guide
Build and maintain Vue.js applications quickly with the standard CLI
- 200 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Vue CLI 3 Quick Start Guide
Build and maintain Vue.js applications quickly with the standard CLI
About This Book
Build Vue apps the right way using Vue CLI 3. Understand how the building blocks of Vue CLI 3 work including npm, webpack, babel, eslint, plugins, GUI, testing, and SCSS. Import third-party libraries and maintain your project.
Key Features
- Learn to work with Vue CLI 3 both on the command line and with a GUI
- Manage VueJS apps, settings, Vue plugins, and third-party libraries
- Learn how to build Vue apps from scratch using webpack, babel, ES6, vue-router, Jest, Cypress, SCSS, and Git
Book Description
The sprawling landscape of various tools in JavaScript web development is becoming overwhelming. This book will show you how Vue CLI 3 can help you take back control of the tool chain. To that end, we'll begin by configuring webpack, utilizing HMR, and using single-file.vue components. We'll also use SCSS, ECMAScript, and TypeScript. We'll unit test with Jest and perform E2E testing with Cypress.
This book will show you how to configure Vue CLI as your default way of building Vue projects. You'll discover the reasons behind using webpack, babel, eslint, and other modern JavaScript toolchain technologies. You'll learn about the inner workings of each through the lens of Vue CLI 3. We'll explore the extendibility of Vue CLI with the built-in settings, and various core and third-party plugins.
Vue CLI helps you work with Vue components, routers, directives, and services in the Vue ecosystem. While learning these concepts, you'll examine the evolution of JavaScript. You'll learn about use of npm, IIFEs, modules in JavaScript, Common.js modules, task runners, npm scripts, module bundlers, and webpack. You'll get familiar with the reasons why Vue CLI 3 is set up the way it is. You'll also learn to perform linting with ESLint and Prettier.
Towards the end, we'll introduce you to working with styles and SCSS. Finally, we'll show you how to deploy your very own Vue project on Github Pages.
What you will learn
- Work with nvm, install Node.js and npm, use Vue CLI 3 with no configuration, via the command line and the graphical user interface
- Build a Vue project from scratch using npm and webpack, and learn about hot module replacement
- Work with Babel settings, configurations, and presets
- Work with Vue plugins, including testing plugins such as Jest and Cypress
- Write, run, and watch unit and E2E tests using TDD assertions in the red-green-refactor cycle
- Work with Vue router and use, nested, lazy-loading, and dynamic routes
- Add SCSS to your projects and work with third-party Vue plugins
- Deploy your Vue apps to Github Pages
Who this book is for
This book is for existing web developers and developers who are new to web development. You must be familiar with HTML, CSS, and JavaScript programming. Basic knowledge of the command line will be helpful but is not necessary.
Frequently asked questions
Information
Webpack in Vue CLI 3
- The evolution of the JavaScript (JS) language from the script tag to module bundlers
- The script tag
- Immediately Invoked Function Expressions (IIFEs), what problems they solve, and what problems they don't
- How Node Package Manager (NPM) helps teams share third-party libraries in their code
- The role of JS task runners and NPM scripts
- What the CommonJS specification is and how modules work in JavaScript and Node.js
- What module bundlers are and how they bridge the gap between Node.js and the browser
- What webpack, and how it works
- How to run webpack on a project
- Bundling assets with webpack using production and development modes
- Adding a Vue project via NPM and using webpack with it
The evolution of the JS language
- The script tag as the answer to adding interactivity to web pages
- Immediately invoked function expressions as the answer to modularizing libraries and avoiding code collisions
- The problem with IIFEs
- Sharing third-party libraries in a team environment with NPM
- JS task runners and NPM scripts
- Modules in JS
The script tag
Immediately Invoked Function Expressions
The problem with IIFEs
Sharing third-party libraries in a team environment with NPM
npm init -y
{
"name": "vue-from-npm",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" &&am...
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- About Packt
- Contributors
- Preface
- Introducing Vue CLI 3
- Webpack in Vue CLI 3
- Babel in Vue CLI 3
- Testing in Vue CLI 3
- Vue CLI 3 and Routing
- Using ESLint and Prettier in Vue CLI 3
- Improving CSS with SCSS
- Deploying Vue CLI 3 Apps on GitHub Pages
- Other Books You May Enjoy